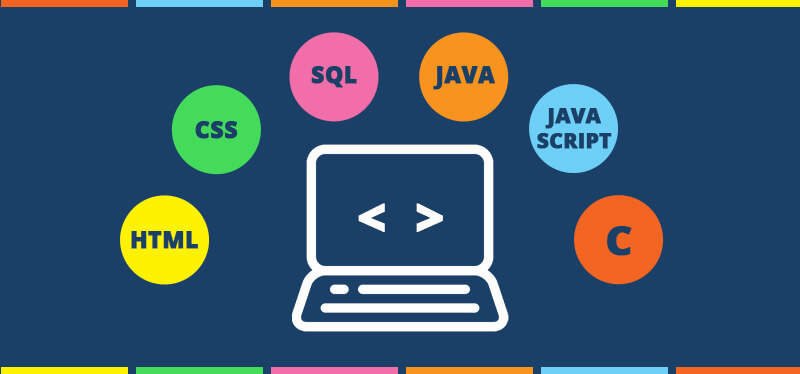
- By Ahsan 03-Jun-2023
- 578
What are Important casting would really know as a .Net Developer?
What are Important casting would really know as a .Net Developer?
As a .NET developer, there are several important casting techniques you should be familiar with. Here are some of the key casting techniques used in C#:
1. Implicit Casting:
Implicit casting, also known as widening conversion, occurs when you assign a value of a smaller data type to a larger data type. The casting is done automatically by the compiler, as it is considered safe. For example:
```csharp
int num = 10;
long bigNum = num; // Implicit casting from int to long
```
2. Explicit Casting:
Explicit casting, also known as narrowing conversion, is required when you assign a value of a larger data type to a smaller data type. Explicit casting requires you to use the cast operator or the `Convert` class. However, be cautious as it may result in data loss or an exception if the value cannot be safely represented in the target type. For example:
```csharp
double bigNum = 3.14;
int num = (int)bigNum; // Explicit casting from double to int
```
3. Boxing and Unboxing:
Boxing is the process of converting a value type (such as `int`, `float`, etc.) to an object type (such as `object`) by encapsulating it within a reference type. Unboxing, on the other hand, is the reverse process of extracting the value type from an object. Boxing and unboxing come with a performance cost, so it's important to use them judiciously. Here's an example:
```csharp
int num = 42;
object boxedNum = num; // Boxing
int unboxedNum = (int)boxedNum; // Unboxing
```
4. Type Conversion Methods:
The .NET framework provides several type conversion methods in the `Convert` class to convert between different data types. These methods are useful when dealing with strings, parsing user input, or converting values from one type to another. Here are a few examples:
string strNum = "42";
int num = Convert.ToInt32(strNum);
double dblNum = 3.14;
string strDblNum = Convert.ToString(dblNum);
5. `as` Operator:
The `as` operator is used for safe type casting, especially when working with reference types. It attempts to cast an object to a specified type and returns `null` if the cast fails, instead of throwing an exception. For example:
```csharp
object obj = "Hello";
string str = obj as string;
if (str != null)
{
// Cast succeeded
}
These are some of the important casting techniques you should be familiar with as a .NET developer. Understanding and correctly using these casting techniques will help you manipulate and convert data between different types effectively in your .NET applications.
In C#, there are several casting functions and operators available to perform type conversions. Here are some of the commonly used casting functions and operators:
1. Direct Casting:
The most common way to perform casting in C# is by using parentheses and the target type. This is known as direct casting or explicit casting. For example:
```csharp
int intValue = 10;
double doubleValue = (double)intValue; // Casting int to double
```
2. Convert Class Methods:
The `Convert` class in the .NET framework provides various methods for converting one type to another. These methods are helpful when converting between different data types, particularly when dealing with strings. Some commonly used methods include `ToInt32()`, `ToDouble()`, `ToString()`, etc. Example:
```csharp
string strValue = "42";
int intValue = Convert.ToInt32(strValue); // String to int conversion
```
3. Parse Methods:
Most primitive types and some framework types provide `Parse()` methods that allow you to convert strings into the corresponding types. These methods are useful when you have a string representation of a value and want to convert it into the appropriate data type. Example:
```csharp
string strValue = "3.14";
double doubleValue = double.Parse(strValue); // String to double conversion
```
4. `as` Operator:
The `as` operator is used for safe type casting when working with reference types. It attempts to cast an object to a specified type and returns `null` if the cast fails, instead of throwing an exception. Example:
```csharp
object obj = "Hello";
string str = obj as string;
if (str != null)
{
// Cast succeeded
}
```
5. `is` Operator:
The `is` operator is used to check if an object is of a specific type. It returns a Boolean value indicating whether the object can be cast to the specified type. Example:
```csharp
object obj = "Hello";
if (obj is string)
{
// Object is of type string
}
These are some of the casting functions and operators available in C# that allow you to convert values between different types. It's important to choose the appropriate casting technique based on the specific conversion scenario and handle any potential exceptions that may occur during the casting process.