how to implement chatgpt completions in asp.net mvc
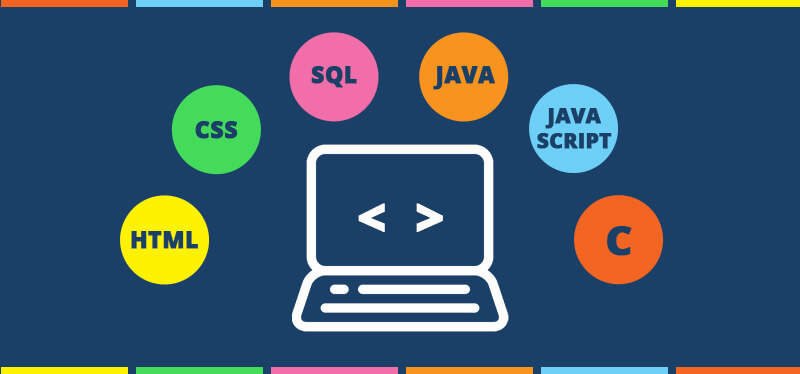
- By Junaid A September-26-2023
- 221
Side hustle
here is the sample code to implement chatgpt completion
using InnerException.Common.AI.ChatGPT;
using InnerException.Common.AI.Google;
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Threading.Tasks;
using System.Web;
using System.Web.Mvc;
namespace InnerException.WebUI.Controllers
{
public class ChatGPTController : Controller
{
// GET: ChatGPT
public ActionResult Index()
{
var myDeserializedClass = new RootChat();
return View(myDeserializedClass);
}
[HttpPost]
public async Task<ActionResult> Index(string kw)
{
var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Post, "https://api.openai.com/v1/chat/completions");
request.Headers.Add("Authorization", "Bearer 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");
var content = new StringContent("{\r\n \"model\": \"gpt-3.5-turbo\",\r\n \"messages\": [\r\n {\r\n \"role\": \"system\",\r\n \"content\": \"You are a helpful assistant.\"\r\n },\r\n {\r\n \"role\": \"user\",\r\n \"content\": \""+kw+"\"\r\n }\r\n ]\r\n }", null, "application/json");
System.Net.ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls11 | SecurityProtocolType.Tls12;
request.Content = content;
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
var r = await response.Content.ReadAsStringAsync();
// Console.WriteLine(response.Content);
var myDeserializedClass = JsonConvert.DeserializeObject<RootChat>(r);
return View(myDeserializedClass);
}
[HttpPost]
public async Task<ActionResult> SendFile(string kw)
{
var client = new HttpClient();
var request = new HttpRequestMessage(HttpMethod.Post, "https://api.openai.com/v1/chat/completions");
request.Headers.Add("Authorization", "Bearer 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");
var content = new StringContent("{\r\n \"model\": \"gpt-3.5-turbo\",\r\n \"messages\": [\r\n {\r\n \"role\": \"system\",\r\n \"content\": \"You are a helpful assistant.\"\r\n },\r\n {\r\n \"role\": \"user\",\r\n \"content\": \"" + kw + "\"\r\n }\r\n ]\r\n }", null, "application/json");
System.Net.ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls11 | SecurityProtocolType.Tls12;
request.Content = content;
var response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
var r = await response.Content.ReadAsStringAsync();
// Console.WriteLine(response.Content);
var myDeserializedClass = JsonConvert.DeserializeObject<RootChat>(r);
return View(myDeserializedClass);
}
public class RootFileobj
{
public string id { get; set; }
public string @object { get; set; }
public int bytes { get; set; }
public int created_at { get; set; }
public string filename { get; set; }
public string purpose { get; set; }
public string status { get; set; }
public object status_details { get; set; }
}
public async Task<ActionResult> ShareFileAsync()
{
string apiKey = "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";
string apiUrl = "https://api.openai.com/v1/files";
string filePath = "E:\\TO_Do_list.txt"; // "mydata.jsonl"; // Provide the correct file path
// filePath = "{\"path\":\"E:\\TO_Do_list.txt\",\"foo\":\"bar\"}";
RootFileobj o = new RootFileobj();
o.id = "";
// o.object= "";
using (HttpClient client = new HttpClient())
{
System.Net.ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls11 | SecurityProtocolType.Tls12;
// Set the authorization header
client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Bearer", apiKey);
// Create a MultipartFormDataContent
using (MultipartFormDataContent content = new MultipartFormDataContent())
{
// Add the "purpose" field
content.Add(new StringContent("fine-tune"), "purpose");
string filedata = "";
content.Add(new StringContent("file"), filedata);
// Add the file
using (FileStream fileStream = new FileStream(filePath, FileMode.Open))
{
var fileContent = new StreamContent(fileStream);
fileContent.Headers.ContentDisposition = new ContentDispositionHeaderValue("form-data")
{
Name = "file",
FileName = Path.GetFileName(filePath)
};
content.Add(fileContent);
// Send the POST request
HttpResponseMessage response = await client.PostAsync(apiUrl, content);
// Check the response
if (response.IsSuccessStatusCode)
{
string responseContent = await response.Content.ReadAsStringAsync();
Console.WriteLine("Response: " + responseContent);
}
else
{
Console.WriteLine("Error: " + response.StatusCode);
}
}
}
}
return View();
}
}
}