How to Implement Area Chart in asp.net mvc
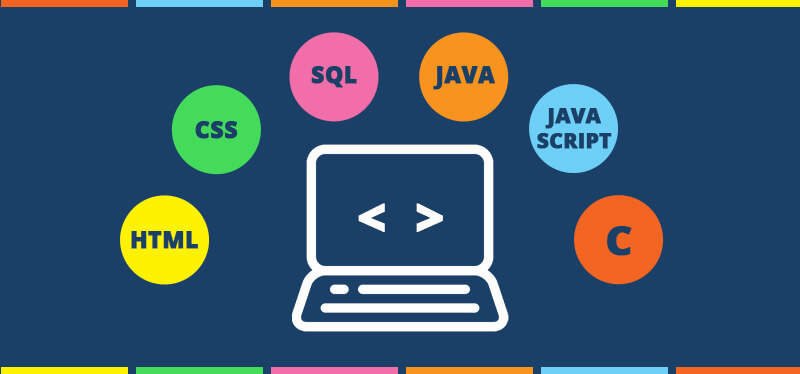
- By Junaid A February-10-2018
- 4090
Literature
Data visualization is an essential aspect of modern web applications, allowing users to understand complex information quickly. One popular type of chart for displaying data trends over time is the Area Chart. In this article, we will explore how to implement an Area Chart in an ASP.NET MVC application using the Chart.js library.
Prerequisites
Before we begin, make sure you have the following prerequisites in place:
Visual Studio or Visual Studio Code for ASP.NET MVC development.
An ASP.NET MVC project set up.
Step 1: Create an ASP.NET MVC Project
If you haven't already, create a new ASP.NET MVC project in Visual Studio. You can choose the "Empty" template or any other template that suits your needs.
Step 2: Install Chart.js
To use the Chart.js library in your ASP.NET MVC project, you need to install it via npm. Open a terminal window and navigate to your project directory. Run the following command to install Chart.js:
npm install chart.js --save
This command will download and install the Chart.js library and add it to your project's dependencies.
Step 3: Create a Controller and View
Next, create a controller and view for displaying the Area Chart. In Visual Studio, right-click on the "Controllers" folder, select "Add," and choose "Controller." Name it something like "ChartController."
Now, create a view for your controller action. Right-click on the "Views" folder, create a new folder called "Chart" (or any name you prefer), and inside that folder, create a new view called "AreaChart.cshtml."
Step 4: Configure the Chart Data
In your controller, create an action method that will retrieve and provide data to the view. For demonstration purposes, let's assume you have data representing a time series.
public class ChartController : Controller
{
public IActionResult AreaChart()
{
// Replace this with your data retrieval logic
var labels = new List<string> { "January", "February", "March", "April", "May" };
var data = new List<int> { 10, 20, 15, 30, 25 };
ViewBag.ChartLabels = labels;
ViewBag.ChartData = data;
return View();
}
}
Step 5: Create the Area Chart in the View
In your "AreaChart.cshtml" view, you can create the Area Chart using JavaScript and the Chart.js library. Here's an example of how to do this:
@{
var chartLabels = ViewBag.ChartLabels as List<string>;
var chartData = ViewBag.ChartData as List<int>;
}
<canvas id="areaChart" width="400" height="200"></canvas>
@section scripts {
<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>
<script>
var ctx = document.getElementById('areaChart').getContext('2d');
var areaChart = new Chart(ctx, {
type: 'line',
data: {
labels: @Html.Raw(Json.Serialize(chartLabels)),
datasets: [{
label: 'Data',
data: @Html.Raw(Json.Serialize(chartData)),
backgroundColor: 'rgba(75, 192, 192, 0.2)',
borderColor: 'rgba(75, 192, 192, 1)',
borderWidth: 1,
fill: true,
}]
},
options: {
scales: {
y: {
beginAtZero: true
}
}
}
});
</script>
}
This code does the following:
Retrieves the chart labels and data from the ViewBag.
Creates an HTML canvas element for the chart.
Includes the Chart.js library.
Configures the chart using JavaScript, specifying the chart type as 'line' for an Area Chart.
Step 6: Render the Chart
Finally, you need to ensure that your action method in the controller renders the "AreaChart" view when accessed. This can be achieved by returning the view as shown in the controller code in Step 4.
Step 7: Run the Application
Build and run your ASP.NET MVC application. Navigate to the URL associated with your "AreaChart" action, and you should see a beautiful Area Chart displaying your data.
Congratulations! You have successfully implemented an Area Chart in your ASP.NET MVC application using the Chart.js library. You can further customize the chart by exploring the Chart.js documentation and adjusting the chart options to fit your specific needs.