Useful code for ASP .Net Developer
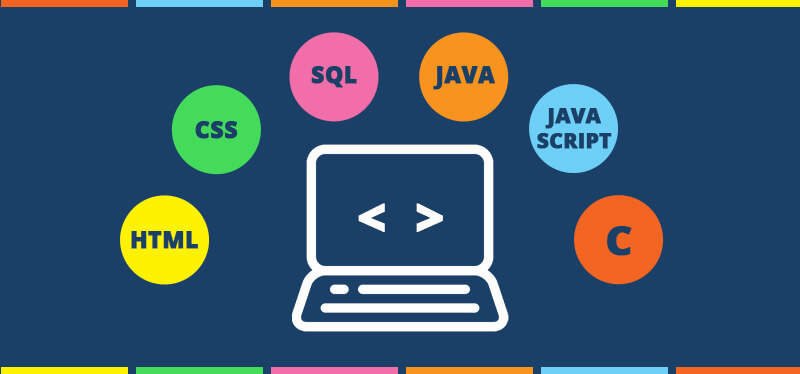
- By Junaid A February-24-2023
- 263
Literature
public class BusinessManager
{
//InnerException.Common.BusinessManager.
//InnerException.Common.BusinessManager.FMShare()
public static string FMShare()
{
return "https://futureminutes.com/fm-share.png";
}
Calculate Posted Month From now
public static string GetPostedMonth(DateTime postedDateTime)
{
string msg = string.Empty;
var crrentDateTime = DateTime.Now;
var postedDateTimeTemap = DateTime.Now.AddMinutes(-8);
DateTime.TryParse(postedDateTime.ToString(), out postedDateTimeTemap);
var diff = crrentDateTime.Subtract(postedDateTimeTemap);
msg = CultureInfo.CurrentCulture.DateTimeFormat.GetAbbreviatedMonthName(postedDateTime.Month)+" " + postedDateTime.ToString("dd");
// msg = postedDateTime.ToString("MM dd");
return msg.ToString();
}
Calculate Posted Time
public static string GetPosteTime(DateTime postedDateTime)
{
string msg = string.Empty;
var crrentDateTime = DateTime.Now;
var postedDateTimeTemap = DateTime.Now.AddMinutes(-8);
DateTime.TryParse(postedDateTime.ToString(), out postedDateTimeTemap);
var diff = crrentDateTime.Subtract(postedDateTimeTemap);
msg = postedDateTime.ToString("hh:mm tt");
return msg.ToString();
}
public static string GetPostedTime(DateTime postedDateTime)
{
string msg = string.Empty;
var crrentDateTime = DateTime.Now;
var postedDateTimeTemap = DateTime.Now.AddMinutes(-8);
DateTime.TryParse(postedDateTime.ToString(), out postedDateTimeTemap);
var diff = crrentDateTime.Subtract(postedDateTimeTemap);
if (diff.Days >= 365)
{
msg = diff.Days / 365 + " years ago";
}
else if (diff.Days >= 30)
{
msg = diff.Days / 30 + " month ago";
}
else if (diff.Days > 0)
{
msg = diff.Days.ToString() + " days ago";
}
else if (diff.Hours > 0)
{
msg = diff.Hours.ToString() + " hours ago";
}
else if (diff.Minutes > 0)
{
msg = diff.Minutes.ToString() + " minuts ago";
}
else
{
msg = diff.Seconds.ToString() + " seconds ago";
}
return msg;
}
Generate Random Number:
public static string GetRandomNumber(int length)
{
var rnd = new Random();
const string charPool = "1234567890";
var rs = new StringBuilder();
while (length-- > 0)
rs.Append(charPool[(int)(rnd.NextDouble() * charPool.Length)]);
return rs.ToString();
}
public static string Base64Encode(string plainText)
{
var plainTextBytes = System.Text.Encoding.UTF8.GetBytes(plainText);
return System.Convert.ToBase64String(plainTextBytes);
}
public static string Base64Decode(string base64EncodedData)
{
var base64EncodedBytes = System.Convert.FromBase64String(base64EncodedData);
return System.Text.Encoding.UTF8.GetString(base64EncodedBytes);
}
Get Path of the Project
public static string FullyQualifiedApplicationPath
{
get
{
//Return variable declaration
var appPath = string.Empty;
if (System.Web.HttpContext.Current.Session[SessionKeyItems.DomainPath] != null)
{
appPath = System.Web.HttpContext.Current.Session[SessionKeyItems.DomainPath].ToString();
}
else
{
//Getting the current context of HTTP request
var context = System.Web.HttpContext.Current;
//Checking the current context content
if (context != null)
{
//Formatting the fully qualified website url/name
appPath = string.Format("{0}://{1}{2}{3}",
context.Request.Url.Scheme,
context.Request.Url.Host,
context.Request.Url.Port == 80
? string.Empty
: ":" + context.Request.Url.Port,
context.Request.ApplicationPath);
}
if (!appPath.EndsWith("/"))
appPath += "/";
System.Web.HttpContext.Current.Session[SessionKeyItems.DomainPath] = appPath;
}
return appPath;
}
}
public static bool SendEmail(ref EmailMessageObject emailmessage)
{
MailMessage mailmessage = new MailMessage();
if (emailmessage.FromName != string.Empty)
{
try
{
emailmessage.FromEmail = emailmessage.FromName + " <" + emailmessage.FromEmail + ">";
}
catch (Exception ex)
{
Console.Write(ex.ToString());
}
}
mailmessage.From = new MailAddress(emailmessage.FromEmail);
foreach (string mailto in emailmessage.MailTo)
{
if (mailto != string.Empty)
{
mailmessage.To.Add(new MailAddress(mailto));
}
}
//cc email
if (emailmessage.CCEmail != null)
{
foreach (string mailtocc in emailmessage.CCEmail)
{
if (mailtocc != string.Empty)
{
mailmessage.CC.Add(new MailAddress(mailtocc));
}
}
}
//bcc email
if (emailmessage.BCCEmail != null)
{
foreach (string mailtobcc in emailmessage.BCCEmail)
{
if (mailtobcc != string.Empty)
{
mailmessage.Bcc.Add(new MailAddress(mailtobcc));
}
}
}
mailmessage.Subject = emailmessage.Subject;
mailmessage.Body = emailmessage.Body;
mailmessage.IsBodyHtml = true;
string smtp = ConfigurationSettings.AppSettings["SmtpHostName"].ToString(); // System.Configuration.ConfigurationManager.AppSettings["SMTP"].ToString();
// string smtp = "relay-hosting.secureserver.net";
SmtpClient smtpmail = new SmtpClient(smtp);
smtpmail.UseDefaultCredentials = false;
// smtpmail.Host = ConfigurationManager.AppSettings["HOSTNAME"].ToString();
smtpmail.Port = Convert.ToInt32(ConfigurationSettings.AppSettings["SmtpPort"].ToString());//Convert.ToInt32(ConfigurationManager.AppSettings["SMTPPORT"].ToString());
string username = ConfigurationSettings.AppSettings["SmtpUserName"].ToString();
string password = ConfigurationSettings.AppSettings["SmtpPassword"].ToString();
smtpmail.Credentials = new System.Net.NetworkCredential(username.ToString(), password.ToString());
// Boolean EnableSsl = Convert.ToInt32(ConfigurationSettings.AppSettings["SmtpPort"].ToString());//Convert.ToInt32(ConfigurationManager.AppSettings["SMTPPORT"].ToString());
smtpmail.EnableSsl = false;
try
{
smtpmail.Send(mailmessage); // Disable Email for testing
}
catch (Exception ex)
{
Console.Write(ex.ToString());
}
return true;
}
Get Date and Time
public static DateTime DateTimeNow()
{
TimeZone curTimeZone = TimeZone.CurrentTimeZone;
DateTime curUTC = curTimeZone.ToUniversalTime(DateTime.Now.AddHours(5));
return curUTC;
}
}
}
Truncate Function:
public static string TruncateSimple(string input, int length)
{
string retstr = "";
if (!string.IsNullOrEmpty(input))
{
if (input.Length <= length)
{
return retstr = input;
}
else
{
retstr = input.Substring(0, length);
return retstr;
}
}
return retstr;
}