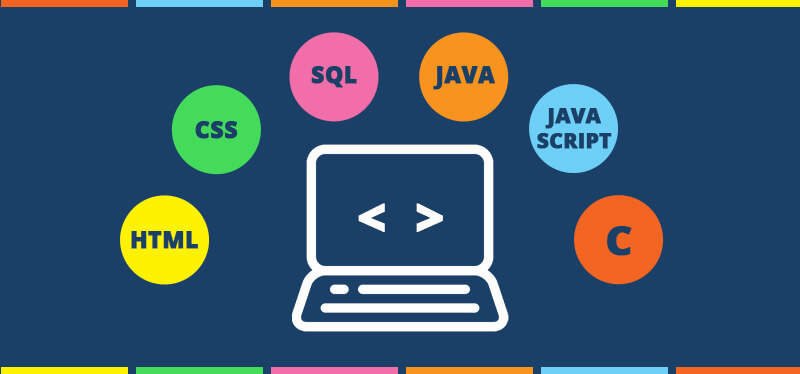
How we can do CRUD in jQuery
CRUD stands for Create, Read, Update, and Delete, and it refers to the basic operations that we can perform on data. In this article, we will learn how to use jQuery, a popular JavaScript library, to do CRUD operations on an HTML table that displays some products.
Create:
To create a new product and add it to the table, we need to use the jQuery.ajax() method to send a PUT request to the server with the product data. The server will then return a response with the product ID and other information. We can use the jQuery.append() method to append a new table row with the product data to the table body.
For example, suppose we have an HTML form with input fields for product name, introduction date, and URL. We can use the following jQuery code to create a new product when the form is submitted:
// get the form element
var form = $("#productForm");
// attach a submit event handler
form.submit(function(event) {
// prevent the default form submission
event.preventDefault();
// get the form data as an object
var productData = form.serializeObject();
// send a PUT request to the server with the product data
$.ajax({
url: "/products",
type: "PUT",
data: productData,
dataType: "json",
success: function(data) {
// get the product ID from the server response
var productId = data.id;
// create a new table row with the product data
var productRow = $("<tr>")
.append($("<td>").text(productData.name))
.append($("<td>").text(productData.date))
.append($("<td>").text(productData.url))
.append($("<td>").text(productId));
// append the new row to the table body
$("#productTable tbody").append(productRow);
},
error: function(error) {
// handle any error from the server
alert(error.responseText);
}
});
});
Read:
To read the existing products from the server and display them in the table, we need to use the jQuery.ajax() method to send a GET request to the server. The server will then return a response with an array of products. We can use the jQuery.each() method to loop through each product and append a table row with the product data to the table body.
For example, suppose we have an empty HTML table with a header row for product name, introduction date, URL, and ID. We can use the following jQuery code to read and display all products when the page is loaded:
// run this code when the document is ready
$(document).ready(function() {
// send a GET request to the server for all products
$.ajax({
url: "/products",
type: "GET",
dataType: "json",
success: function(data) {
// loop through each product in the response array
$.each(data, function(index, product) {
// create a new table row with the product data
var productRow = $("<tr>")
.append($("<td>").text(product.name))
.append($("<td>").text(product.date))
.append($("<td>").text(product.url))
.append($("<td>").text(product.id));
// append the new row to the table body
$("#productTable tbody").append(productRow);
});
},
error: function(error) {
// handle any error from the server
alert(error.responseText);
}
});
});
Update:
To update an existing product and modify its data in the table, we need to use the jQuery.ajax() method to send a POST request to the server with the product ID and the updated data. The server will then return a response with a confirmation message. We can use the jQuery.find() and jQuery.text() methods to find and update the corresponding table cell with the new data.
For example, suppose we have an HTML form with input fields for product name, introduction date, and URL, and a hidden input field for product ID. We can use the following jQuery code to update an existing product when the form is submitted:
// get the form element
var form = $("#productForm");
// attach a submit event handler
form.submit(function(event) {
// prevent the default form submission
event.preventDefault();
// get the form data as an object
var productData = form.serializeObject();
// get the product ID from the hidden input field
var productId = productData.id;
// send a POST request to the server with the product ID and updated data
$.ajax({
url: "/products/" + productId,
type: "POST",
data: productData,
dataType: "json",
success: function(data) {
// get the confirmation message from the server response
var message = data.message;
// find the table row that matches the product ID
var productRow = $("#productTable tbody tr").filter(function() {
return $(this).find("td:last").text() == productId;
});
// update each table cell with the new data
productRow.find("td:eq(0)").text(productData.name);
productRow.find("td:eq(1)").text(productData.date);
productRow.find("td:eq(2)").text(productData.url);
// display a success message
alert(message);
},
error: function(error) {
// handle any error from the server
alert(error.responseText);
}
});
});
Delete:
To delete an existing product and remove it from the table, we need to use the jQuery.ajax() method to send a DELETE request to the server with the product ID. The server will then return a response with a confirmation message. We can use the jQuery.remove() method to remove the corresponding table row from the table body.
For example, suppose we have an HTML button for each product that allows us to delete it. We can use the following jQuery code to delete an existing product when its button is clicked:
// attach a click event handler for each delete button
$(".deleteButton").click(function(event) {
// prevent any default action for this button click
event.preventDefault();
// get the button element that was clicked
var button = $(this);
// get the product ID from its data attribute
var productId = button.data("id");
// send a DELETE request to the server with the product ID
$.ajax({
url: "/products/" + productId,
type: "DELETE",
dataType: "json",
success: function(data) {
// get confirmation message from server response
var message = data.message;
// find and remove table row that matches this button's parent row
button.closest("tr").remove();
// display success message
alert(message);
},
error: function(error) {
// handle any error from server
alert(error.responseText);
}
});
});
Conclusion:
In this article, we have learned how to use jQuery to do CRUD operations on an HTML table that displays some products. We have used jQuery.ajax() method to send different types of requests (PUT, GET, POST, DELETE) to communicate with our server-side API. We have also used various jQuery methods (append(), each(), find(), text(), remove(), etc.) to manipulate our HTML elements based on our data.