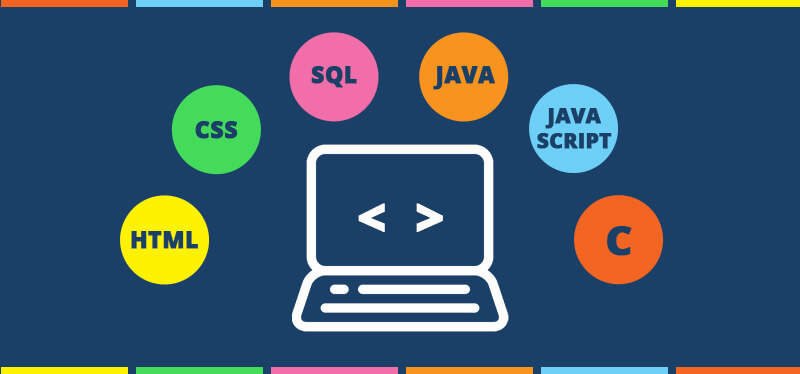
In MVC, you can get the checkbox values generated by a foreach loop by following these steps:
1. In your View, use a foreach loop to generate the checkboxes. Make sure to assign a unique name and value to each checkbox.
`***********************Html******************************
@foreach (var item in Model)
{
<input type="checkbox" name="selectedItems" value="@item.Id" /> @item.Name
}
************************************************************
2. In your Controller, define an action method that handles the form submission.
******************************C Sharp***************************
[HttpPost]
public ActionResult SubmitForm(List<int> selectedItems)
{
// Process the selectedItems
// ...
return View();
}
************************************************************
3. In the action method, the parameter `selectedItems` should be of type `List<int>`, which will automatically bind to the selected checkbox values.
4. When the form is submitted, the selected checkbox values will be passed to the `SubmitForm` action method as a list of integers. You can then process the selected items as required.
Note: Ensure that the form submission method is set to "POST" in the View, and the `SubmitForm` action method is decorated with `[HttpPost]` attribute.
By following these steps, you will be able to retrieve the selected checkbox values generated by the foreach loop in MVC.
Another Example:
Suppose you have a model called `Item` with the properties `Id` and `Name`. You want to generate checkboxes for a list of items in your View and retrieve the selected checkbox values in your Controller.
1. Create a model class `Item.cs`:
******************************csharp******************************
public class Item
{
public int Id { get; set; }
public string Name { get; set; }
}
*********************************************************************
2. In your Controller, create an action method that returns the View with a list of items:
******************************csharp******************************
public ActionResult Index()
{
List<Item> items = new List<Item>
{
new Item { Id = 1, Name = "Item 1" },
new Item { Id = 2, Name = "Item 2" },
new Item { Id = 3, Name = "Item 3" }
};
return View(items);
}
************************************************************
3. Create a View called `Index.cshtml` with the following code:
******************************html******************************
@model List<Item>
@using (Html.BeginForm("SubmitForm", "YourControllerName", FormMethod.Post))
{
for (int i = 0; i < Model.Count; i++)
{
<input type="checkbox" name="selectedItems" value="@Model[i].Id" /> @Model[i].Name<br />
}
<input type="submit" value="Submit" />
}
************************************************************
4. In your Controller, create an action method that handles the form submission:
******************************csharp******************************
[HttpPost]
public ActionResult SubmitForm(List<int> selectedItems)
{
// Process the selectedItems
foreach (int itemId in selectedItems)
{
// Perform the desired operations using the selected item's Id
// ...
}
return View();
}
************************************************************
5. When the form is submitted, the selected checkbox values will be passed to the `SubmitForm` action method as a list of integers. You can then process the selected items as required.
Make sure to replace "YourControllerName" with the actual name of your Controller in the View's `Html.BeginForm` method.
This example demonstrates how to generate checkboxes for a list of items using a foreach loop in MVC and retrieve the selected checkbox values in the Controller.