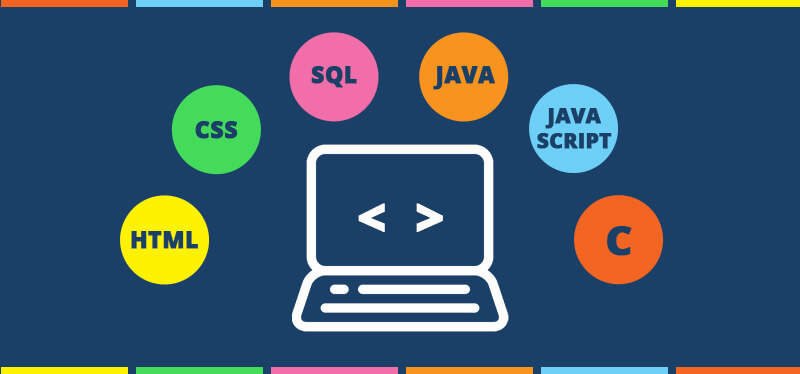
- Asp.Net Core
- 834
- November-08-2023
- by Ehtisham
In recent years, conversational AI has become increasingly popular, allowing businesses and developers to engage with users in a more interactive and natural way. OpenAI's GPT-3, and its variants like ChatGPT, have revolutionized the field of natural language processing and made it possible to build advanced chatbots and virtual assistants. In this article, we will explore how to use ASP.NET Core, a popular web framework by Microsoft, to create a web application that leverages ChatGPT for conversational AI.
Prerequisites
Before you get started, make sure you have the following prerequisites in place:
-
ASP.NET Core: You need to have ASP.NET Core installed on your development machine. You can download it from the official ASP.NET Core website.
-
OpenAI API Key: You should have an API key from OpenAI to access ChatGPT. You can sign up for access on the OpenAI platform.
-
Visual Studio or Visual Studio Code: You can choose your preferred development environment. Visual Studio and Visual Studio Code are both excellent choices for ASP.NET Core development.
Setting Up Your ASP.NET Core Project
-
Create a New ASP.NET Core Project: Start by creating a new ASP.NET Core project using the
dotnet new
command or your development environment. You can choose a Web API or MVC project template based on your requirements. -
Install the OpenAI SDK: To interact with ChatGPT, you'll need the OpenAI .NET SDK. You can install it using NuGet with the following command:
dotnet add package OpenAI
-
Configure OpenAI API Key: In your
Startup.cs
file, configure the OpenAI API key. You can use theIConfiguration
interface to store your API key securely in app settings or environment variables.using Microsoft.Extensions.Configuration; using OpenAI; public void ConfigureServices(IServiceCollection services) { // Load API key from configuration var apiKey = Configuration["OpenAI:ApiKey"]; // Configure OpenAI API client services.AddSingleton(new OpenAIApi(apiKey)); }
-
Create a Controller for ChatGPT: Create a controller in your ASP.NET Core project to handle incoming chat requests and communicate with ChatGPT.
using Microsoft.AspNetCore.Mvc; using OpenAI; [ApiController] [Route("api/chat")] public class ChatController : ControllerBase { private readonly OpenAIApi _openAIApi; public ChatController(OpenAIApi openAIApi) { _openAIApi = openAIApi; } [HttpPost] public async Task<IActionResult> Chat([FromBody] ChatRequest request) { try { var response = await _openAIApi.ChatCompletion.Create(request); return Ok(new { response.choices[0].message.content }); } catch (Exception ex) { return BadRequest(new { error = ex.Message }); } } }
Building the Frontend
-
Create a Chat Interface: You can use HTML, CSS, and JavaScript to create a chat interface on your frontend. This can be a simple chatbox where users enter text, and the chat responses are displayed.
-
Handle User Input: When a user enters a message, make an API call to your ASP.NET Core backend to interact with ChatGPT. You can use JavaScript's
fetch
or any library like Axios for this purpose. -
Display Chat Responses: Once you receive a response from your backend, display it in the chat interface.
Deploying Your Application
Finally, deploy your ASP.NET Core application to a web server or a cloud platform of your choice. Ensure that your API key is securely stored in your production environment, and follow best practices for securing your application.
By combining ASP.NET Core with ChatGPT, you can build powerful conversational AI applications that can assist users, answer questions, and provide personalized experiences. This integration allows you to leverage the capabilities of OpenAI's ChatGPT within the robust and scalable ASP.NET Core framework.