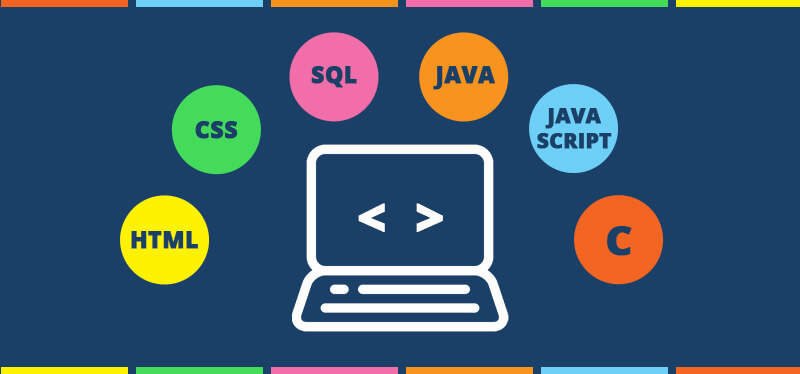
How we can deserialize xml response of the API in C#?
Certainly! Let's dive deeper into the process of deserializing an XML response in C# using the `XmlSerializer` class.
1. Define the class structure:
In order to deserialize the XML response, you need to create a class that represents the structure of the XML. The class should have properties that correspond to the elements in the XML. You can use various attributes to customize the mapping between XML elements and class properties. Here's an example:
-----------------Csharp--------------------
[XmlRoot("RootElement")]
public class ApiResponse
{
[XmlElement("Name")]
public string Name { get; set; }
[XmlElement("Age")]
public int Age { get; set; }
}
-------------------------------------------------
In this example, the `[XmlRoot]` attribute specifies the root element name of the XML, and the `[XmlElement]` attributes map the properties to their corresponding XML elements.
2. Deserialize the XML response:
Once you have the class defined, you can use the `XmlSerializer` class to perform the deserialization. Here's how:
-------------------csha----------------------
using System.Xml.Serialization;
// Assuming you have the XML response stored in a string variable called 'xmlResponse'
XmlSerializer serializer = new XmlSerializer(typeof(ApiResponse));
using (StringReader reader = new StringReader(xmlResponse))
{
ApiResponse result = (ApiResponse)serializer.Deserialize(reader);
}
```
In this code snippet, you create an instance of the `XmlSerializer` class, specifying the type of the object you want to deserialize (`typeof(ApiResponse)`). Then, you create a `StringReader` object to read from the `xmlResponse` string. The `Deserialize` method of the `XmlSerializer` class is used to perform the actual deserialization, and it returns an object of type `ApiResponse`.
3. Access the deserialized data:
After the deserialization is complete, you can access the data from the XML response through the properties of the deserialized object. For example:
```csharp
string name = result.Name;
int age = result.Age;
```
Here, `result` is an instance of the `ApiResponse` class that contains the deserialized data. You can access the properties of `result` just like any other object properties.
It's important to handle any exceptions that may occur during the deserialization process. For example, an `InvalidOperationException` can be thrown if the XML structure doesn't match the class structure, and an `XmlException` can be thrown if there are errors in the XML format.
By following these steps,we effectively deserialize an XML response from an API in C# and access the data in a structured manner using the class properties.