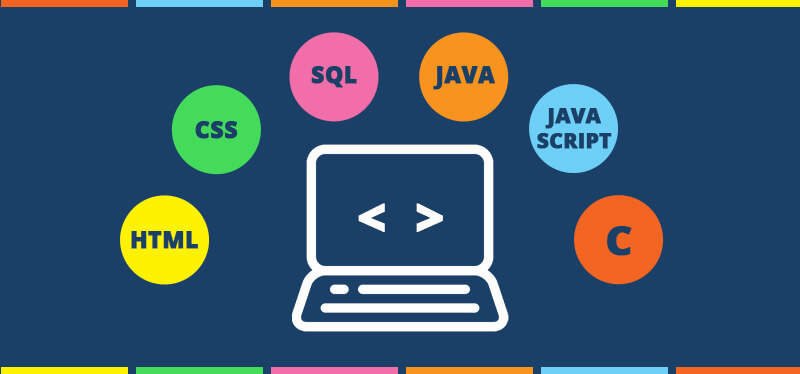
- .Net
- 667
- June-09-2018
- by
Keep Session Live using cookie in asp.net mvc.
Create a static class
1)
public static class CookieItemKeys
{public const string CookieObjectName = "CookieObjectName";
public const string UserType = "UserType";
public const string UserAccountID = "UserAccountID";
public const string Name = "Name";
}
2 - Create a function that will save the cookie, we will pass a model to that function
private void RememberMe(UserAccount login) // save data in cookies
{
var userInfo = new HttpCookie(CookieItemKeys.CookieObjectName);userInfo[CookieItemKeys.Name] = login.FirstName;
userInfo[CookieItemKeys.UserAccountID] = login.UserAccountID.ToString();
userInfo[CookieItemKeys.UserType] = login.UserAccountID.ToString();
userInfo.Expires = DateTime.Today.AddDays(Convert.ToInt32(365));
Response.Cookies.Add(userInfo);
}
3- Create A function that will return cookies those were saved
public UserAccount CheckCookies()
{
var u = new UserAccount();
HttpCookie reqCookies = Request.Cookies[CookieItemKeys.CookieObjectName];
if (reqCookies != null)
{
u.FirstName = reqCookies[CookieItemKeys.Name];
u.UserAccountID = Convert.ToInt32(CookieItemKeys.UserAccountID);
u.UserType = reqCookies[CookieItemKeys.UserType];
}
return u;
}
4- Create a BaseController that will check the cookie and set data in session
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using SaudiPhone.Common;
using System.Configuration;
using System.Text;
using System.Collections.Generic;
using System.Linq;
using System.Text.RegularExpressions;
using SaudiPhone.EF;
using System.Web.Mvc;namespace SaudiPhone.WebUIV2.Controllers
{
public class MyBaseController : ActionFilterAttribute
{
public override void OnActionExecuting(ActionExecutingContext filterContext)
{
if (filterContext.HttpContext.Session[SessionItemKeys.UserProfileId] == null)
{var u = new UserAccount();
var context = filterContext.HttpContext;
HttpCookie reqCookies = context.Request.Cookies[CookieItemKeys.CookieObjectName];
if (reqCookies != null)
{
saudiphonedbEntities db = new saudiphonedbEntities();u.FirstName = reqCookies[CookieItemKeys.Name];
u.UserAccountID = Convert.ToInt32(reqCookies[CookieItemKeys.UserAccountID]);
u.UserType = reqCookies[CookieItemKeys.UserType];var userModel = db.UserAccounts.FirstOrDefault(w => w.UserAccountID == u.UserAccountID);
if (userModel != null)
{
context.Session[SessionItemKeys.UserProfileId] = userModel.UserAccountID;
context.Session[SessionItemKeys.UserTypeId] = userModel.UserType;
context.Session[SessionItemKeys.UserAccountId] = userModel.UserAccountID;
context.Session[SessionItemKeys.ContactName] = userModel.FirstName + " " + userModel.LastName;
}}
}
}
}
}
5- Create a file FilterConfig.cs mostly it is arleady availbe in a project
and register filter as
using System.Web;
using System.Web.Mvc;
using SaudiPhone.WebUIV2;
using SaudiPhone.WebUIV2.Controllers;namespace SaudiPhone.WebUIV2
{
public class FilterConfig
{
public static void RegisterGlobalFilters(GlobalFilterCollection filters)
{
filters.Add(new HandleErrorAttribute());
filters.Add(new MyBaseController());}
}
}