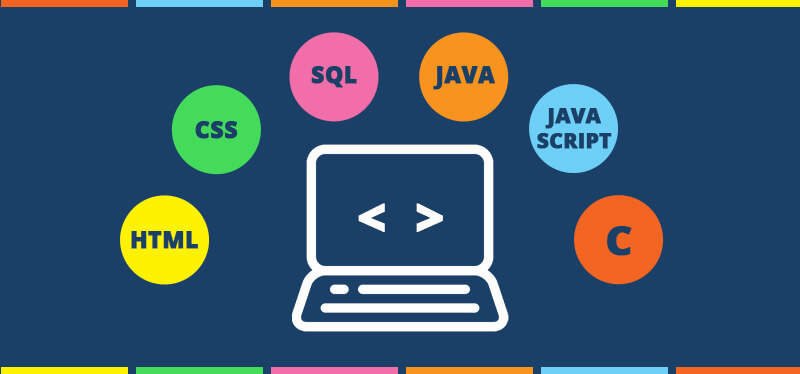
Best practice to create CRUD in asp.net MVC 5 using jQuery
- Java Script
- HTML
- C# Code
Java Script Code
function AddDiagnosis(id) {
if (id > 0) {
$.get("/Diagnosis/CrudDiagnosis", { id: id }, function (data) {
App.unblockUI($("#boxDiagnosis"));
// $("#box").html(data);
});
////}
}
else { //Screen for Add
$.get("/Diagnosis/CrudDiagnosis", { id: id }, function (data) {
// App.unblockUI($("#box"));
$("#boxDiagnosis").html(data);
});
}
};
function BindDiagnosis()
{
$.get("../../Diagnosis/ListDiagnosis", function (data) {
// App.unblockUI('#box');
$("#boxDiagnosis").html(data);
});
}
$("#CancelDiagnosis").unbind('click').bind('click', function () {
BindDiagnosis();
});
// Purpose:CRUD operations
// Created on:
// Author: Asif Jans.
$(function () {
$("#CrudDiagnosis").unbind('submit').bind("submit", function (e) {
debugger;
e.preventDefault();
var form = $(this);
// var va = form.validate();
//if (form.valid()) {
//App.blockUI('body');
var url = form.attr('action');
$.post(url, form.serialize(), function (data) {
//App.unblockUI("body");
if (data == '') {
// alert("Done");
BindDiagnosis();
}
else {
// start Dispaly error message/warning message in dialog box
alert(data);
//bootbox.dialog({
// title: '<center><b> Warning </b></center>',
// message: '<img src="https://cdn3.iconfinder.com/data/icons/softwaredemo/PNG/24x24/Warning.png"/> <span style="padding-left:10px;">' + data + '</span>',
// buttons: {
// success: { label: "OK", className: "btn-success" }
// }
//});
//App.unblockUI("body");
}
});
}
//}
);
});
HTML
Create html page that must have a div with the Id “boxDiagnosis”
<div id="boxDiagnosis">
</div>
On Html page write java script in
<Script>
- Java script code will be here
</Script> Java script in a file and add reference of that file.
Note! Include jquery refrence in the html page or in layout page
Code of C# in Controller
public ActionResult ListDiagnosis()
{
string AccessTokenKFS = "";
if (Session[KFSOnlineShop.Common.MOADTO.SessionItemKeysV1.AccessTokenAuthV1] != null)
{
AccessTokenKFS = Session[KFSOnlineShop.Common.MOADTO.SessionItemKeysV1.AccessTokenAuthV1].ToString();
}
List<ItechnocratHealthDiabetesPatientDiagnosisDTO> GetItechnocratHealthDiabetesPatientDiagnosis = new List<ItechnocratHealthDiabetesPatientDiagnosisDTO>();
KFSOnlineShop.Common.AuthenticateUserResult p = new KFSOnlineShop.Common.AuthenticateUserResult();
if (Session[KFSOnlineShop.Common.MOADTO.SessionItemKeysV1.SelectedUserProfile]!=null)
{
p = Session[KFSOnlineShop.Common.MOADTO.SessionItemKeysV1.SelectedUserProfile] as KFSOnlineShop.Common.AuthenticateUserResult;
}
GetItechnocratHealthDiabetesPatientDiagnosis = KFSOnlineShop.Common.ItechnocratHealthRepoistoryV1.GetItechnocratHealthDiabetesPatientDiagnosis(p.UserProfileID.ToString(), p.ClinicID.ToString(), p.DoctorsID.ToString(), AccessTokenKFS);
return View(GetItechnocratHealthDiabetesPatientDiagnosis);
}
#region CRUD OPERATIONS
public ActionResult CrudDiagnosis(int id)
{
string AccessTokenKFS = "";
if (Session[KFSOnlineShop.Common.MOADTO.SessionItemKeysV1.AccessTokenAuthV1] != null)
{
AccessTokenKFS = Session[KFSOnlineShop.Common.MOADTO.SessionItemKeysV1.AccessTokenAuthV1].ToString();
}
ItechnocratHealthDiabetesPatientDiagnosisDTO o = new ItechnocratHealthDiabetesPatientDiagnosisDTO();
ViewBag.HealthDiabetesConditionsTypeDetails = KFSOnlineShop.Common.ItechnocratHealthRepoistoryV1.GetItechnocratHealthDiabetesConditionsTypeDetails();
ViewBag.GetItechnocratHealthDiabetesTest = KFSOnlineShop.Common.ItechnocratHealthRepoistoryV1.GetItechnocratHealthDiabetesTest();
// o.diabetesSymptomsDiagnosisID== bind this with proper api
o.patientbloodsugarlevel = 50;
ViewBag.ItechnocratHealthObesitySymptomsDTO = KFSOnlineShop.Common.ItechnocratHealthRepoistoryV1.GetItechnocratHealthObesitySymptoms(AccessTokenKFS);
return View(o);
}
[HttpPost]
public string CrudDiagnosis(ItechnocratHealthDiabetesPatientDiagnosisDTO o)
{
string apiResponsemessage = "";
string AccessTokenKFS = "";
if (Session[KFSOnlineShop.Common.MOADTO.SessionItemKeysV1.AccessTokenAuthV1] != null)
{
AccessTokenKFS = Session[KFSOnlineShop.Common.MOADTO.SessionItemKeysV1.AccessTokenAuthV1].ToString();
}
KFSOnlineShop.Common.AuthenticateUserResult p = new KFSOnlineShop.Common.AuthenticateUserResult();
if (Session[KFSOnlineShop.Common.MOADTO.SessionItemKeysV1.SelectedUserProfile] != null)
{
p = Session[KFSOnlineShop.Common.MOADTO.SessionItemKeysV1.SelectedUserProfile] as KFSOnlineShop.Common.AuthenticateUserResult;
o.PatientID = p.UserProfileID;
o.ClinicID = p.ClinicID;
o.DoctorsID = p.DoctorsID; // logged user id will be as doctor id
}
apiResponsemessage = KFSOnlineShop.Common.ItechnocratHealthRepoistoryV1.CreateItechnocratHealthDiabetesDiagnosis2(o, AccessTokenKFS);
ViewBag.HealthDiabetesConditionsTypeDetails = KFSOnlineShop.Common.ItechnocratHealthRepoistoryV1.GetItechnocratHealthDiabetesConditionsTypeDetails();
ViewBag.GetItechnocratHealthDiabetesTest = KFSOnlineShop.Common.ItechnocratHealthRepoistoryV1.GetItechnocratHealthDiabetesTest();
// o.diabetesSymptomsDiagnosisID== bind this with proper api
ViewBag.ItechnocratHealthObesitySymptomsDTO = KFSOnlineShop.Common.ItechnocratHealthRepoistoryV1.GetItechnocratHealthObesitySymptoms(AccessTokenKFS);
return apiResponsemessage;
}
#endregion
CRUD using jQuery
Call partial view using jQuery