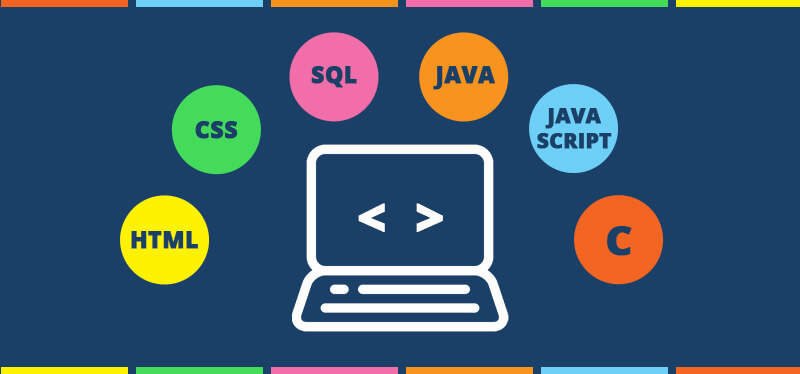
How to send email in asp.net mvc with attachment
Step1
Create a class and set properties those will be used to support email system
[Serializable]
public class EmailMessageObject
{
private string _fromemail;
private string _fromname;
private string[] _mailto;
private string _subject;
private string _body;
private string[] _ccemail;
private string[] _bccemail;
public List<string> AttachmentFiles { get; set; }
public string FromEmail
{
get { return _fromemail; }
set { _fromemail = value; }
}
public string[] CCEmail
{
get { return _ccemail; }
set { _ccemail = value; }
}
public string[] BCCEmail
{
get { return _bccemail; }
set { _bccemail = value; }
}
public string FromName
{
get { return _fromname; }
set { _fromname = value; }
}
public string[] MailTo
{
get { return _mailto; }
set { _mailto = value; }
}
public string Subject
{
get { return _subject; }
set { _subject = value; }
}
public string Body
{
get { return _body; }
set { _body = value; }
}
}
Step 2
Create a function that will send email with some parameters
public static bool SendEmail(EmailMessageObject emailMessage)
{
SmtpClient smtpClient = new SmtpClient("smtp.gmail.com", 587);
NetworkCredential basicCredential = new NetworkCredential("emailid@gmail.com", "pasword");
MailMessage message = new MailMessage();
MailAddress fromAddress = new MailAddress("youremail@gmail.com", "webname");
smtpClient.UseDefaultCredentials = false;
smtpClient.EnableSsl = true;
smtpClient.Credentials = basicCredential;
message.From = fromAddress;
message.Subject = emailMessage.Subject;
//Set IsBodyHtml to true means you can send HTML email.
message.IsBodyHtml = true;
message.Body = emailMessage.Body;
if (emailMessage.AttachmentFiles!=null)
{
foreach (var item in emailMessage.AttachmentFiles)
{
//string attachmentName = item;
System.Net.Mail.Attachment attachment;
attachment = new System.Net.Mail.Attachment(item);
message.Attachments.Add(attachment);
}
}
foreach (var item in emailMessage.MailTo)
{
message.To.Add(item);
}
try
{
smtpClient.Send(message);
return true;
}
catch (Exception ex)
{
return false;
//Error, could not send the message
// Response.Write(ex.Message);
}
}