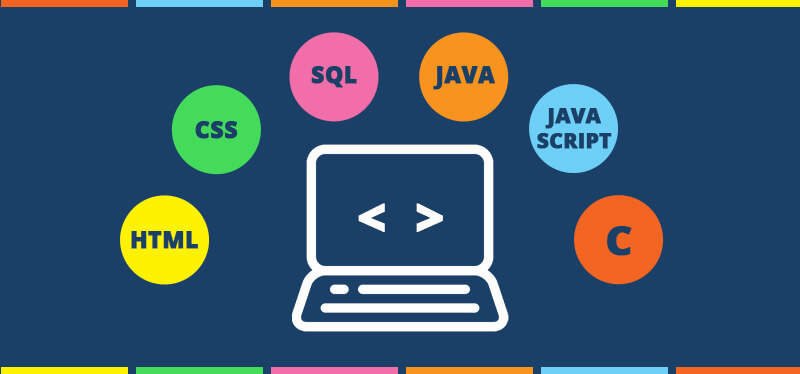
I want to consum api in .net, which client is best and fast to consume api.
several options for consuming APIs in .NET, and the choice of client depends on your specific needs and requirements. Here are some popular choices:
HttpClient: This is a built-in .NET class that provides a simple and easy-to-use way to consume APIs. It supports synchronous and asynchronous requests and provides methods for GET, POST, PUT, DELETE, and other HTTP verbs.
RestSharp: RestSharp is a popular third-party library for consuming RESTful APIs in .NET. It provides a simple and intuitive API for making HTTP requests and deserializing responses into .NET objects.
Refit: Refit is another popular third-party library that simplifies the process of consuming RESTful APIs in .NET. It allows you to define API endpoints as C# interfaces, and it handles all the details of making HTTP requests and deserializing responses.
Flurl: Flurl is a fluent URL builder and HTTP client library for .NET that makes it easy to consume APIs. It provides a simple and expressive syntax for constructing URLs and making HTTP requests.
WebClient: This is another built-in .NET class that provides a simple way to consume APIs. It supports synchronous requests only and provides methods for GET, POST, PUT, DELETE, and other HTTP verbs.
In terms of performance, all of these options are fast and efficient, and the choice of client depends more on your specific needs and preferences than on performance. It's always a good idea to test different options and choose the one that works best for your use case.
which client more fast to consume api
In terms of performance, the built-in HttpClient class is generally considered to be the fastest option for consuming APIs in .NET. This is because it is optimized for performance and provides low-level control over the HTTP request and response, allowing you to fine-tune the performance of your API calls.
That being said, the performance difference between HttpClient and other third-party libraries such as RestSharp, Refit, and Flurl is often negligible, especially for small to medium-sized API calls. The performance of your API calls will also depend on factors such as the size of the payload, network latency, and server response time, which can vary widely.
Therefore, when choosing a client library for consuming APIs in .NET, it's important to consider factors other than just performance, such as ease of use, maintainability, and support for specific features or authentication mechanisms required by the API. Ultimately, the best choice of client library will depend on your specific use case and requirements.
how to call api using HttpClient in C#
To call an API using HttpClient in C#, you can follow these general steps:
Create an instance of the HttpClient class:
arduino
Copy code
HttpClient client = new HttpClient();
Define the request, including the HTTP method, URL, and any headers or query parameters:
csharp
Copy code
string url = "https://api.example.com/users";
string query = "?page=1&per_page=10";
client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
Send the request and retrieve the response:
csharp
Copy code
HttpResponseMessage response = await client.GetAsync(url + query);
string responseBody = await response.Content.ReadAsStringAsync();
Process the response, which may involve parsing the response body, handling errors, or converting the response into a .NET object:
sql
Copy code
if (response.IsSuccessStatusCode)
{
List<User> users = JsonConvert.DeserializeObject<List<User>>(responseBody);
foreach (User user in users)
{
Console.WriteLine("Name: " + user.Name + ", Email: " + user.Email);
}
}
else
{
Console.WriteLine("API call failed with status code: " + response.StatusCode);
}
This is just a basic example, and there are many ways to customize and extend this code depending on your specific needs and the API you are calling. It's also important to handle exceptions and errors properly and follow best practices for managing the HttpClient instance, such as reusing it across multiple requests and disposing of it properly when it's no longer needed.
HttpClient is more fast approch to consume api