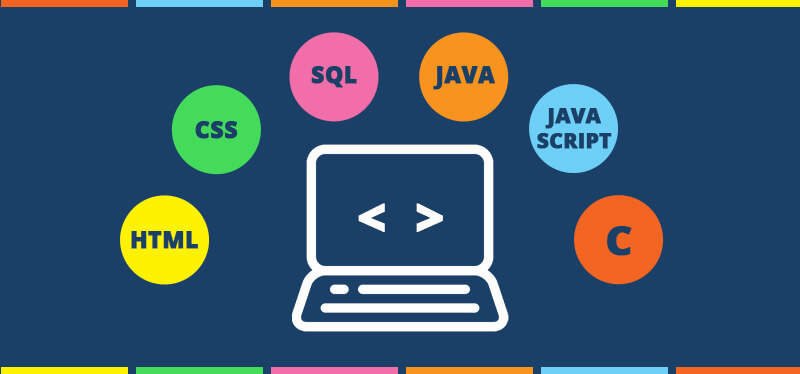
To export data from an inquiry table to an Excel sheet using ASP.NET MVC and C#, you can follow these steps:
Step 1: Create a method in your controller that retrieves the inquiry data from your database or any other source. Let's assume your method is called "GetInquiryData".
----------------------------csharp----------------------------
public ActionResult GetInquiryData()
{
// Retrieve the inquiry data from your source (e.g., database)
var inquiryData = // Retrieve the data and format it as per your requirement
return View(inquiryData);
}
----------------------------------------------------------------------
Step 2: Create a view to display the inquiry data. This view will have a button to trigger the export to Excel functionality. Let's assume your view is called "InquiryDataView".
----------------------------html----------------------------
@model IEnumerable<YourNamespace.InquiryData>
<table>
<tr>
<th>Column 1</th>
<th>Column 2</th>
<!-- Add more columns as per your requirement -->
</tr>
@foreach (var item in Model)
{
<tr>
<td>@item.Column1</td>
<td>@item.Column2</td>
<!-- Add more columns as per your requirement -->
</tr>
}
</table>
<button id="exportBtn">Export to Excel</button>
<script>
document.getElementById('exportBtn').addEventListener('click', function () {
window.location.href = '/ControllerName/ExportToExcel';
});
</script>
------------------------------------------------------------------------------------
Step 3: Create a method in your controller to handle the export functionality. Let's assume your method is called "ExportToExcel".
----------------------------csharp--------------------------------------------------------
public void ExportToExcel()
{
var inquiryData = // Retrieve the inquiry data from your source (e.g., database)
// Create a new Excel package
using (var package = new ExcelPackage())
{
// Create a new worksheet
var worksheet = package.Workbook.Worksheets.Add("InquiryData");
// Set the headers
worksheet.Cells[1, 1].Value = "Column 1";
worksheet.Cells[1, 2].Value = "Column 2";
// Add more headers as per your requirement
// Set the data
int row = 2;
foreach (var item in inquiryData)
{
worksheet.Cells[row, 1].Value = item.Column1;
worksheet.Cells[row, 2].Value = item.Column2;
// Add more columns as per your requirement
row++;
}
// Set the response headers
Response.Clear();
Response.ContentType = "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet";
Response.AddHeader("content-disposition", "attachment;filename=InquiryData.xlsx");
// Write the Excel package to the response stream
Response.BinaryWrite(package.GetAsByteArray());
Response.End();
}
}
------------------------------------------------------------------------------------
Note: Make sure to add the necessary references and namespaces, such as `System.Web.Mvc` and `OfficeOpenXml`, for the above code to work. You may need to install the "EPPlus" NuGet package to work with Excel files.
This implementation uses the EPPlus library to generate the Excel file. The "ExportToExcel" method creates an Excel package, adds a worksheet, populates it with the inquiry data, and writes the package to the response stream. When the user clicks the "Export to Excel" button on the view, it triggers the "ExportToExcel" method, and the generated Excel file will be downloaded by the user.