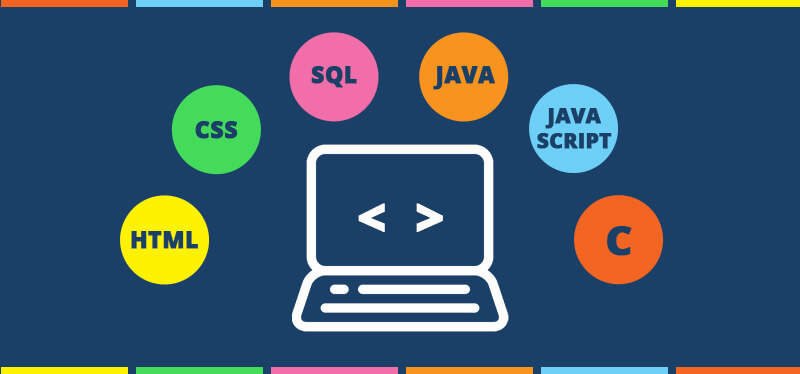
create appointment booking page with available time slot using database ms sql server
To create an appointment booking page with available time slots using MS SQL Server, you can follow these general steps:
- Create a database table to store appointment information, including the date, time, duration, and any other relevant information. Here's an example SQL query to create a basic appointments table:
sqlCopy code
CREATE TABLE Appointments ( ID INT IDENTITY(1,1) PRIMARY KEY, AppointmentDate DATE NOT NULL, StartTime TIME NOT NULL, Duration INT NOT NULL, ClientName VARCHAR(50) NOT NULL, ClientEmail VARCHAR(50) NOT NULL );
- Create a stored procedure to retrieve available time slots for a specific date. This procedure should query the Appointments table to find any existing appointments on the specified date and then generate a list of available time slots. Here's an example stored procedure:
lessCopy code
CREATE PROCEDURE GetAvailableTimeSlots @AppointmentDate DATE AS BEGIN DECLARE @StartTime TIME = '08:00:00' DECLARE @EndTime TIME = '17:00:00' DECLARE @Duration INT = 60 SELECT @StartTime AS StartTime, @EndTime AS EndTime INTO #TimeSlots WHILE @StartTime < DATEADD(MINUTE, -@Duration, @EndTime) BEGIN SET @StartTime = DATEADD(MINUTE, @Duration, @StartTime) IF NOT EXISTS ( SELECT 1 FROM Appointments WHERE AppointmentDate = @AppointmentDate AND StartTime = @StartTime ) BEGIN INSERT INTO #TimeSlots (StartTime, EndTime) VALUES (@StartTime, DATEADD(MINUTE, @Duration, @StartTime)) END END SELECT * FROM #TimeSlots END
-
Create a web page that displays a calendar control and allows users to select a date. When the user selects a date, make an AJAX call to the GetAvailableTimeSlots stored procedure to retrieve a list of available time slots for that date.
-
Display the available time slots on the web page, allowing the user to select a time slot and enter their name and email address.
-
When the user submits the form, insert a new record into the Appointments table with the selected date, time, duration, client name, and client email.
These are just general steps to give you an idea of how to create an appointment booking page with available time slots using MS SQL Server. You'll need to customize the code to fit your specific requirements and design preferences.
display list of available time slot with the interval of 15 minutes
To display a list of available time slots with an interval of 15 minutes, you can modify the stored procedure I provided earlier to increment the start time by 15 minutes instead of 60 minutes. Here's an example modified stored procedure:
CREATE PROCEDURE GetAvailableTimeSlots @AppointmentDate DATE AS BEGIN DECLARE @StartTime TIME = '08:00:00' DECLARE @EndTime TIME = '17:00:00' DECLARE @Duration INT = 15 SELECT @StartTime AS StartTime, @EndTime AS EndTime INTO #TimeSlots WHILE @StartTime < DATEADD(MINUTE, -@Duration, @EndTime) BEGIN SET @StartTime = DATEADD(MINUTE, @Duration, @StartTime) IF NOT EXISTS ( SELECT 1 FROM Appointments WHERE AppointmentDate = @AppointmentDate AND StartTime = @StartTime ) BEGIN INSERT INTO #TimeSlots (StartTime, EndTime) VALUES (@StartTime, DATEADD(MINUTE, @Duration, @StartTime)) END END SELECT * FROM #TimeSlots END
In this modified stored procedure, the @Duration parameter is set to 15 instead of 60, and the @StartTime variable is incremented by 15 minutes in the WHILE loop instead of 60 minutes. This will generate a list of available time slots with a 15-minute interval.
When you display the available time slots on the web page, you can format the time slots to display in a user-friendly format, such as "8:00 AM - 8:15 AM", "8:15 AM - 8:30 AM", and so on.
write code to save appointments in asp.net mvc
To save appointments in ASP.NET MVC, you can follow these general steps:
- Create a model class to represent the appointment data. This class should include properties for the appointment date, start time, duration, client name, and client email address.
csharpCopy code
public class Appointment { public int ID { get; set; } [Required] [Display(Name = "Appointment Date")] public DateTime AppointmentDate { get; set; } [Required] [Display(Name = "Start Time")] public TimeSpan StartTime { get; set; } [Required] public int Duration { get; set; } [Required] [Display(Name = "Client Name")] public string ClientName { get; set; } [Required] [Display(Name = "Client Email")] public string ClientEmail { get; set; } }
- Create a controller to handle the appointment creation process. This controller should include an action method to display the appointment booking form, and another action method to handle the form submission.
csharpCopy code
public class AppointmentsController : Controller { private ApplicationDbContext db = new ApplicationDbContext(); // GET: Appointments/Create public ActionResult Create() { return View(); } // POST: Appointments/Create [HttpPost] [ValidateAntiForgeryToken] public ActionResult Create([Bind(Include = "AppointmentDate,StartTime,Duration,ClientName,ClientEmail")] Appointment appointment) { if (ModelState.IsValid) { db.Appointments.Add(appointment); db.SaveChanges(); return RedirectToAction("Index", "Home"); } return View(appointment); } }
In this example, the controller uses an Entity Framework DbContext to save the appointment data to the database.
- Create a view to display the appointment booking form. This view should include form controls for the appointment date, start time, duration, client name, and client email address.
lessCopy code
@model Appointment @using (Html.BeginForm()) { @Html.AntiForgeryToken() <div class="form-horizontal"> <h4>Appointment</h4> <hr /> @Html.ValidationSummary(true, "", new { @class = "text-danger" }) <div class="form-group"> @Html.LabelFor(model => model.AppointmentDate, htmlAttributes: new { @class = "control-label col-md-2" }) <div class="col-md-10"> @Html.EditorFor(model => model.AppointmentDate, new { htmlAttributes = new { @class = "form-control" } }) @Html.ValidationMessageFor(model => model.AppointmentDate, "", new { @class = "text-danger" }) </div> </div> <div class="form-group"> @Html.LabelFor(model => model.StartTime, htmlAttributes: new { @class = "control-label col-md-2" }) <div class="col-md-10"> @Html.EditorFor(model => model.StartTime, new { htmlAttributes = new { @class = "form-control" } }) @Html.ValidationMessageFor(model => model.StartTime, "", new { @class = "text-danger" }) </div> </div> <div class="form-group"> @Html.LabelFor(model => model.Duration, htmlAttributes: new { @class = "control-label col-md-2" }) <div class="col-md-10"> @Html.EditorFor(model => model.Duration, new { htmlAttributes = new { @class = "form-control" } }) @Html.ValidationMessageFor(model => model.Duration, "", new { @class = "text-danger" }) </div
create a page to show list of appointments, so that user can choose for booking
To create a page that shows a list of appointments for booking, you can follow these steps:
- Create an action method in the AppointmentsController to retrieve a list of appointments from the database and pass them to a view.
csharpCopy code
public ActionResult Index() { var appointments = db.Appointments.ToList(); return View(appointments); }
- Create a view to display the list of appointments. In this view, you can use the Razor syntax to loop through the list of appointments and display them in a table.
phpCopy code
@model List<Appointment> <table class="table"> <thead> <tr> <th>Date</th> <th>Start Time</th> <th>Duration</th> <th>Client Name</th> <th>Client Email</th> <th>Action</th> </tr> </thead> <tbody> @foreach (var appointment in Model) { <tr> <td>@appointment.AppointmentDate.ToShortDateString()</td> <td>@appointment.StartTime.ToString()</td> <td>@appointment.Duration</td> <td>@appointment.ClientName</td> <td>@appointment.ClientEmail</td> <td> @Html.ActionLink("Book Appointment", "Create", "Appointments", new { id = appointment.ID }, null) </td> </tr> } </tbody> </table>
In this example, the Razor syntax is used to loop through the list of appointments and display each appointment's date, start time, duration, client name, and client email address in a table. A link is also included to allow the user to book an appointment.
- Test the page by navigating to the /Appointments URL in your web browser. The list of appointments should be displayed, and the user should be able to book an appointment by clicking the "Book Appointment" link next to an appointment.
Jack Carter
2 hours agoi think that some how, we learn who we really are and then live with that decision, great post!
you can view the more detail via link https://www.youtube.com/watch?v=HpZgwHU1GcIChing xang
2 hours agoi think that some how, we learn who we really are and then live with that decision, great post!
Danial Comb
2 hours agoi think that some how, we learn who we really are and then live with that decision, great post!
Jack Carter
2 hours agoi think that some how, we learn who we really are and then live with that decision, great post!