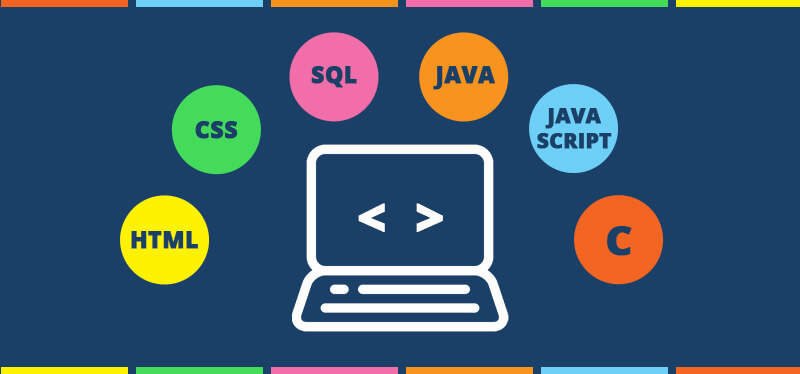
Login with facebook in asp.net mvc
1) Create a Facebook App using http://developers.facebook.com
then get following keys
2) add in web.config
<add key="FacebookAppId" value="383189645425584"/>
<add key="FacebookAppSecret" value="59e9bf6b1d846cc947ab9a70dfc2aa98"/>
3) add this link
<a id="fbLoginContainer" class="hidden-xs" href="/Account/Facebook">Login with fb </a>
4) Add Action in Controller as
Facebook and add nuget of facebookppk
or in command run "Install-Package Facebook -Version 7.0.6"
add this
property
private Uri RediredtUri
{get
{
var uriBuilder = new UriBuilder(Request.Url);
uriBuilder.Query = null;
uriBuilder.Fragment = null;
uriBuilder.Path = Url.Action("FacebookCallback");
return uriBuilder.Uri;
}
}
public ActionResult Facebook()
{
var fb = new FacebookClient();
var loginUrl = fb.GetLoginUrl(new
{
client_id = ConfigurationManager.AppSettings["FacebookAppId"].ToString(),client_secret = ConfigurationManager.AppSettings["FacebookAppSecret"].ToString(),
redirect_uri = RediredtUri.AbsoluteUri,
response_type = "code",
scope = "email"
});
return Redirect(loginUrl.AbsoluteUri);
}
5) Call Back Action URL
public ActionResult FacebookCallback(string code)
{
Boolean isProfileCompleted = false;
try
{
var fb = new FacebookClient();dynamic result = fb.Post("oauth/access_token", new
{
client_id = ConfigurationManager.AppSettings["FacebookAppId"].ToString(),
client_secret = ConfigurationManager.AppSettings["FacebookAppSecret"].ToString(),
redirect_uri = RediredtUri.AbsoluteUri,
code = code
});var accessToken = result.access_token;
Session["AccessToken"] = accessToken;
fb.AccessToken = accessToken;
dynamic me = fb.Get("me?fields=link,first_name,currency,last_name,email,gender,locale,timezone,verified,picture,age_range, birthday");
string email = me.email;
//TempData["email"] = me.email;
//TempData["first_name"] = me.first_name;
//TempData["lastname"] = me.last_name;
//TempData["picture"] = me.picture.data.url;
//TempData["age"] = me.age_range;
FormsAuthentication.SetAuthCookie(email, false);if (!_uow.Accounts.Get().Any(a => a.EmailAddress == me.email))
{#region new Account
var account = new Account();
account.FirstName = me.first_name;
account.LastName = me.last_name;
account.IsEmailVerified = true;
account.IsProfileCompleted = false;account.IsActive = true;
account.IsDeleted = false;
account.Channel = WebAccessChannelEnum.Web.ToString();
account.CreatedDate = BusinessManager.DateTimeNow();
account.EmailAddress = me.email;
account.Gender = me.gender;
account.Balance = 0;
account.AccountType_Id = (int)EAccountType.BUYER;
var a = _uow.Accounts.SignUp(account);
SessionItems.Add(SessionKey.ACCOUNT, a);
SendWelcomeEmail(a);Session["CreateedAccount"] = a;
#endregion
// will be used on next screen to show confirmaiton message
}
else
{
Account a = _uow.Accounts.AccountByEmail(me.email);
isProfileCompleted = Convert.ToBoolean(a.IsProfileCompleted);
SessionItems.Add(SessionKey.ACCOUNT, a);
Session["CreateedAccount"] = a; // will be used on next screen to show confirmaiton messagevar a =
}
if (isProfileCompleted==false)
{
SessionItems.Add(SessionKey.ISPROFILE_COMPLETED, false);
return RedirectToAction("Index", "EditProfile");
}if (SessionItems.Get(SessionKey.PREVIOUS_URL) != null)
{
string prev_URL = SessionItems.Get(SessionKey.PREVIOUS_URL).ToString();
return Redirect(BusinessManager.FullyQualifiedApplicationPath((int)WebAccessChannelEnum.Web) + prev_URL);
//return RedirectToAction(view, controller);}
else
{
TempData["fc"] = "true";return RedirectToAction("Index", "Home", new { feature = "feature" });
}
// return RedirectToAction("ThankYou");
}catch
{
TempData["errorMsg"] = "Your account is suspended, please contact to admin.";
// it will show user is not active , please contact admin
return RedirectToAction("SignIn");
}}
thats done. on call back get Contact Detail and save in database.