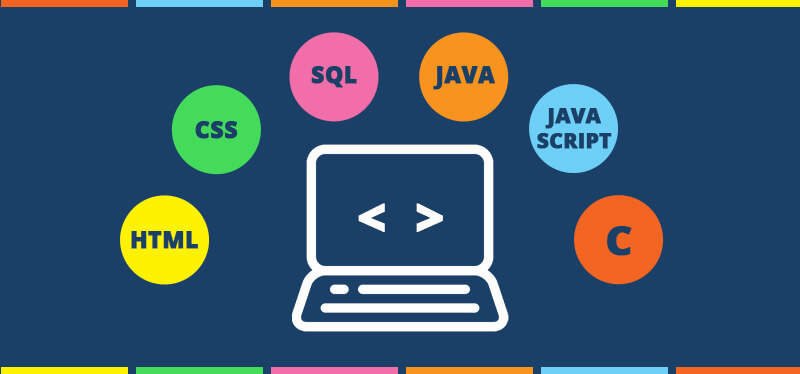
How to content negotiation in web api ?
media type formatter in Web API is represented by an abstract class called MediaTypeFormatter.
Web API framework comes with the following formatters by default.
System.Net.Http.Formatting.JsonMediaTypeFormatter
System.Net.Http.Formatting.XmlMediaTypeFormatter
System.Net.Http.Formatting.FormUrlEncodedMediaTypeFormatter
System.Web.Http.ModelBinding.JQueryMvcFormUrlEncodedFormatter
Web API comes up with the following media type mappings by default.
QueryStringMapping
UriPathExtensionMapping
RequestHeaderMapping
MediaRangeMapping
To build you custom media type mapping, you would need to create a class that extends the MediaTypeMapping as shown in the code snippet below.
public class IDGMediaTypeMapping : MediaTypeMapping
{
protected override double OnTryMatchMediaType(HttpResponseMessage response)
{
//Write your custom code here
}
}
The following code snippet illustrates how you can retrieve the names of all supported formatters in Web API by iterating the HttpConfiguration.Formatters collection.
[HttpGet]
public List<string> GetAllFormatters()
{
List<string> lstFormaters = new List<string>();
foreach (var formatter in this.Configuration.Formatters)
{
lstFormaters.Add(formatter.GetType().Name);
}
return lstFormaters;
}
Let's now explore how we can work with content negotiation to select the formatter we want and retrieve the content in the format we need. Consider the following entity class.
***
public class CustomerDTO
{
public Int32 Id
{ get; set; }
public string FirstName
{ get; set; }
public string LastName
{ get; set; }
public string Address
{ get; set; }
}
**
Next, assume that you have a method that populates data into a list of type CustomerDTO and returns it.
private List<CustomerDTO> GetCustomerData()
{
List<CustomerDTO> lstCustomers = new List<CustomerDTO>();
CustomerDTO customer = new CustomerDTO();
customer.Id = 1;
customer.FirstName = "Joydip";
customer.LastName = "Kanjilal";
customer.Address = "Hyderabad, India";
lstCustomers.Add(customer);
return lstCustomers;
}
The following Web API method shows how you can return HttpResponseMessage as a response from your Web API method based on the default content negotiation mechanism available.
[HttpGet]
public HttpResponseMessage GetCustomers()
{
List<CustomerDTO> lstCustomers = GetCustomerData();
IContentNegotiator negotiator = Configuration.Services.GetContentNegotiator();
ContentNegotiationResult result = negotiator.Negotiate(typeof(CustomerDTO), Request, Configuration.Formatters);
return new HttpResponseMessage()
{
Content = new ObjectContent<IEnumerable<CustomerDTO>>(lstCustomers, result.Formatter, result.MediaType.MediaType)
};
}
**