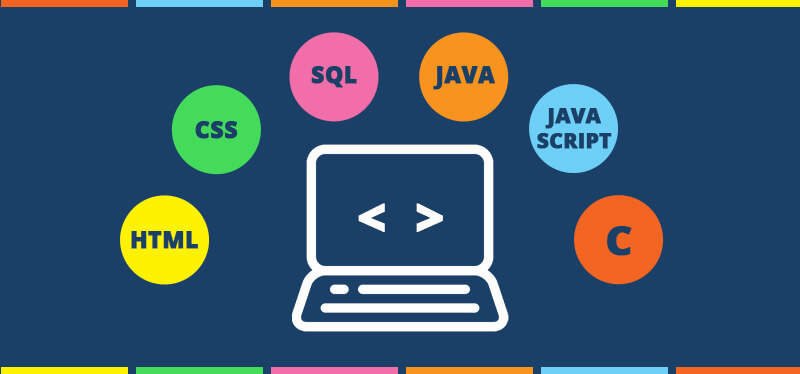
find folder path in sub domain or find js css path in sub domain
in html layout page
@{
var cssjsPath = BusinessManager.FullyQualifiedApplicationPath(); // get deoplyed dommain path
}
<script src="@Url.Content(cssjsPath + "/Scripts/jquery-2.1.4.js")"></script>
Get domain path in java script
<script>
var domainURL = '@(cssjsPath)'; // Get Domain URL and pass it to java script functions// alert(domainURL);
</script>
code in business Manager
using System;
using System.Configuration;
using System.Globalization;
using System.Linq;
using System.Linq.Expressions;
using System.Web;
//using SendGrid;
using System.Collections.Generic;
using System.Drawing;
using System.Net;
using System.Net.Mail;
using System.Text;
using System.IO;
using Myapp.Common.ENUM;
using Myapp.DTO.Users.UserGroup;
using Myapp.DTO.Users;
using Newtonsoft.Json;
using Newtonsoft.Json.Converters;
using Myapp.Common;namespace Myapp.Common
{
/// <summary>
/// Summary description for BusinessManager
/// </summary>
public static class BusinessManager
{public static string LocalTimeToUTCUsingOffset(string dateTime)
{
float gmtoffset = 0;
string utcDateTime = string.Empty;try
{
if (System.Web.HttpContext.Current.Session[SessionItemKeys.GmtOffset] != null)
float.TryParse(System.Web.HttpContext.Current.Session[SessionItemKeys.GmtOffset].ToString(), out gmtoffset);// string dateTime = "11/15/2017 7:00 PM";
DateTime datetimeObj = new DateTime();
DateTime.TryParse(dateTime, out datetimeObj);
datetimeObj = datetimeObj.AddHours(-gmtoffset);
utcDateTime = BusinessManager.ToUtcFormat(datetimeObj);
utcDateTime = utcDateTime.Replace(".000Z", "");
}
catch (Exception ex)
{
}
return utcDateTime;// 11/15/2017 12:00:00 PM
}public static DateTime UTCToLocalUsingOffset(DateTime expiryDatetime)
{
float gmtoffset = 0;
string utcDateTime = string.Empty;
// var dtl = BusinessManager.ToLocalFormat("2017-11-16T02:00:00");try
{
if (System.Web.HttpContext.Current.Session[SessionItemKeys.GmtOffset] != null)
float.TryParse(System.Web.HttpContext.Current.Session[SessionItemKeys.GmtOffset].ToString(), out gmtoffset);
DateTime datetimeObj = new DateTime();DateTime.TryParse(expiryDatetime.ToString(), out datetimeObj);
expiryDatetime = datetimeObj.AddHours(+gmtoffset);
}
catch (Exception)
{throw;
}
// 2018-11-22T21:00:00"//// string expdate = dtl;
//DateTime dt1 = new DateTime();//DateTime.TryParse(dtl.ToString(), out dt1);
//DateTime fdate = dt1.AddHours(+gmtoffset);// 11/15/2017 12:00:00 PM
return expiryDatetime;
}public static string FullyQualifiedApplicationPath3()
{//Return variable declaration
string appPath = null;//Getting the current context of HTTP request
HttpContext context = HttpContext.Current;//Checking the current context content
if (context != null)
{
//Formatting the fully qualified website url/name
appPath = string.Format("{0}://{1}{2}{3}",
context.Request.Url.Scheme,
IpToHostName3(),
context.Request.Url.Port == 80 ? string.Empty : ":" + context.Request.Url.Port,
context.Request.ApplicationPath);
}appPath = appPath.TrimEnd('/');
return appPath;
}
public static string FullyQualifiedApplicationPath()
{
//Return variable declaration
string appPath = null;//Getting the current context of HTTP request
HttpContext context = HttpContext.Current;
if (ConfigurationManager.AppSettings[SessionItemKeys.DomainName] !=null && ConfigurationManager.AppSettings[SessionItemKeys.DomainName].ToString().Length > 5) // check if key is enalbed
{
appPath = ConfigurationManager.AppSettings[SessionItemKeys.DomainName].ToString();
}
else if (System.Web.HttpContext.Current.Session[SessionItemKeys.DomainFullPath] == null)
{//Checking the current context content
if (context != null)
{
//Formatting the fully qualified website url/name
appPath = string.Format("{0}://{1}{2}{3}",
context.Request.Url.Scheme,
IpToHostName(),
context.Request.Url.Port == 80 ? string.Empty : ":" + context.Request.Url.Port,
context.Request.ApplicationPath);
}appPath = appPath.TrimEnd('/');
System.Web.HttpContext.Current.Session[SessionItemKeys.DomainFullPath] = appPath;
}
else
{
appPath = System.Web.HttpContext.Current.Session[SessionItemKeys.DomainFullPath].ToString();
}
return appPath;}
public static string IpToHostName3()
{
HttpContext context = HttpContext.Current;
string IPAdd = GetHostNameOnly();// Request.Url.Host.ToString();// "10.144.87.16";Request.Url.Host.ToString();if (context.Request.Url.Host.ToString().Contains("localhost")==false)
{
IPHostEntry hostEntry = Dns.GetHostEntry(IPAdd);
return hostEntry.HostName;
}
else
{return context.Request.Url.Host;
}
}
public static string IpToHostName()
{string IPAdd = GetHostNameOnly();// Request.Url.Host.ToString();// "10.144.87.16";Request.Url.Host.ToString();
if (IPAdd== "10.144.87.16")
{
IPHostEntry hostEntry = Dns.GetHostEntry(IPAdd);
return hostEntry.HostName;
}
else
{HttpContext context = HttpContext.Current;
return context.Request.Url.Host;}
}public static string GetHostNameOnly()
{//Return variable declaration
string appPath = null;//Getting the current context of HTTP request
HttpContext context = HttpContext.Current;//Checking the current context content
if (context != null)
{
//Formatting the fully qualified website url/nameappPath = context.Request.Url.Host;
}return appPath;
}
public static string FullyQualifiedApplicationPath2()
{
//Return variable declaration
string appPath = null;//Getting the current context of HTTP request
HttpContext context = HttpContext.Current;//Checking the current context content
if (context != null)
{
//Formatting the fully qualified website url/name
appPath = string.Format("{0}://{1}{2}{3}",
context.Request.Url.Scheme,
context.Request.Url.Host,
context.Request.Url.Port == 80 ? string.Empty : ":" + context.Request.Url.Port,
context.Request.ApplicationPath);
}return appPath;
}
public static string ReturnDomainURL()
{
var dommainPath = "";
MemoryCache mycache = new MemoryCache();
if (mycache.Contains(CacheItemKeys.DomainURL))
{
dommainPath = mycache.Get<string>(CacheItemKeys.DomainURL);
}dommainPath= dommainPath + "/Home/Login?sessionTimeOut=timeoutPopup&previousPageUrl=";
// public static string LoginpageUrl = WebConfigurationManager.AppSettings["DomainName"] + "/Home/Login?sessionTimeOut=timeout&previousPageUrl=";
//
return dommainPath;
}public static string FullyQualifiedApplicationPath1()
{
//Return variable declaration http://demo.ivr.com/IVRWebPortal/Home/Logout
var domainURLWithPath = "Domain path is not decteted.";
MemoryCache mycache = new MemoryCache();
if (ConfigurationManager.AppSettings[SessionItemKeys.DomainName] != null && ConfigurationManager.AppSettings[SessionItemKeys.DomainName].ToString().Length > 5) // check if key is enalbed
{
domainURLWithPath = ConfigurationManager.AppSettings[SessionItemKeys.DomainName].ToString();}
else if (mycache.Contains(CacheItemKeys.DomainURL))
{
domainURLWithPath = mycache.Get<string>(CacheItemKeys.DomainURL);
}
else if (System.Web.HttpContext.Current.Session[SessionItemKeys.DomainFullPath] != null)
{
if (mycache.Contains(CacheItemKeys.DomainURL))
{
domainURLWithPath = mycache.Get<string>(CacheItemKeys.DomainURL);
}
else
{
domainURLWithPath = System.Web.HttpContext.Current.Session[SessionItemKeys.DomainFullPath].ToString();}
//int indexofSlash = domainURLWithPath.LastIndexOf("//");
//if (indexofSlash > 5)
//{
// var aStringBuilder = new StringBuilder(domainURLWithPath);
// aStringBuilder.Remove(indexofSlash, 2);
// aStringBuilder.Insert(indexofSlash, "/");
// domainURLWithPath = aStringBuilder.ToString();// domainURLWithPath = domainURLWithPath.Replace("Home/Logout","");
// System.Web.HttpContext.Current.Session[SessionItemKeys.DomainFullPath] = domainURLWithPath;
//}
//char[] MyChar = { '/' };
//domainURLWithPath = domainURLWithPath.TrimEnd(MyChar);
//System.Web.HttpContext.Current.Session[SessionItemKeys.DomainFullPath] = domainURLWithPath;}
// domainURLWithPath = "https://ws.ekit.com/IvrwebPortal";
return domainURLWithPath;
}public static string NumbersCommaCeparator(int value)
{
string valueFormatting = "";
valueFormatting = string.Format("{0:N0}", value);
return valueFormatting;}
public static string BalancePrecisionFormatting(decimal value)
{
string valueFormatting = "";
if (HttpContext.Current.Session[SessionItemKeys.BalancePrecision] != null)
{
valueFormatting = string.Format("{0:N" + HttpContext.Current.Session[SessionItemKeys.BalancePrecision].ToString() + "}", value);
}
return valueFormatting;
}public static string KendoGridAmmountFormatting()
{
if (HttpContext.Current.Session[SessionItemKeys.BalancePrecision] != null)
return "{0:n" + HttpContext.Current.Session[SessionItemKeys.BalancePrecision].ToString() + "}";
return "";
}
public static string BalancePrecisionFormatting(double value)
{
string valueFormatting = "";
if (HttpContext.Current.Session[SessionItemKeys.BalancePrecision]!=null)
{
valueFormatting = string.Format("{0:N" + HttpContext.Current.Session[SessionItemKeys.BalancePrecision].ToString() + "}", value);
}
return valueFormatting;
}
public static Boolean ShowChartLabel()
{
var showChartLabel = false;
if (HttpContext.Current.Session[SessionItemKeys.ClientScreenWidth] != null)
{
int screenWidth = Convert.ToInt32(HttpContext.Current.Session[SessionItemKeys.ClientScreenWidth].ToString());
if (screenWidth > 5440)//show donut charts in one row else 2 rows
{
showChartLabel = true;
}
}
return showChartLabel;}
public static int GetScreenX()
{
int screenWidth = 0;
if (HttpContext.Current.Session[SessionItemKeys.ClientScreenWidth]!=null)
{
screenWidth=Convert.ToInt32((HttpContext.Current.Session[SessionItemKeys.ClientScreenWidth]));
}if (screenWidth <= 320)
{
screenWidth = 50;
}
else if (screenWidth <= 375)
{
screenWidth = 40;
}
else if (screenWidth <= 460)
{
screenWidth = 35;
}
else if (screenWidth <= 768)
{
screenWidth = 10;
}
else if (screenWidth == 1080)
{
screenWidth = 5;
}
else if (screenWidth == 1160)
{
screenWidth = 0;
}
else if (screenWidth == 1366)
{
screenWidth = 0;
}
else if (screenWidth == 1920)
{
screenWidth = -10;
}
else if (screenWidth == 2560)
{
screenWidth = 0;
}
else
{
screenWidth = 0;}
return screenWidth;}
public static Boolean LoggingEnabled()
{
bool enabled = ConfigurationManager.AppSettings["APIErrorLogging"].ToString() == "true";
return enabled;
}public static DateTime GetTimeZoneOffset()
{
TimeZone curTimeZone = TimeZone.CurrentTimeZone;
DateTime curUTC = curTimeZone.ToUniversalTime(DateTime.Now);
return curUTC;
}
public static string UserIPAddress()
{
string ipAddressString = HttpContext.Current.Request.UserHostAddress;if (ipAddressString == null)
return null;IPAddress ipAddress;
IPAddress.TryParse(ipAddressString, out ipAddress);// If we got an IPV6 address, then we need to ask the network for the IPV4 address
// This usually only happens when the browser is on the same machine as the server.
if (ipAddress.AddressFamily == System.Net.Sockets.AddressFamily.InterNetworkV6)
{
ipAddress = System.Net.Dns.GetHostEntry(ipAddress).AddressList
.First(x => x.AddressFamily == System.Net.Sockets.AddressFamily.InterNetwork);
}return ipAddress.ToString();
}
public static string UserIPAddressOld()
{
string ipAddress = "127.0.0.1"; var detectedIp = IPAddressDetection("https://jsonip.com");if (!string.IsNullOrEmpty(detectedIp))
{
ipAddress = detectedIp;
}
else
{
detectedIp = IPAddressDetection("https://api.ipify.org/?format=json");
if (!string.IsNullOrEmpty(detectedIp))
ipAddress = detectedIp;
}
return ipAddress;
}public static string IPAddressDetection(string apiUrl)
{
string ipAddress = string.Empty;
try
{
WebClient web = new WebClient();
UserLocation userLocation = new UserLocation();
System.Net.WebClient wc = new System.Net.WebClient();
string apiResponse = "";apiResponse = wc.DownloadString(apiUrl);
userLocation = JsonConvert.DeserializeObject<UserLocation>(apiResponse);
if (userLocation != null)
ipAddress = userLocation.ip;}
catch (Exception)
{
}
return ipAddress;
}public static bool IsMobileDevice()
{return HttpContext.Current.Request.Browser.IsMobileDevice;
}public static bool ActionAdjustmenRequied()
{
bool actionFormatting = true;
int screenWidthValue=0;int.TryParse(HttpContext.Current.Session[SessionItemKeys.ClientScreenWidth].ToString(), out screenWidthValue);
if (screenWidthValue<2700)
{
actionFormatting= false;
}return actionFormatting;
}
public static UserRightActionsDto ActionRightsReport()
{
return new UserRightActionsDto
{
BulkEdit = true,
Create = true,
Delete = true,
Edit = true,
View = true
};
}
public static UserRightActionsDto ActionRights(int userRightId)
{
if (userRightId == Convert.ToInt32(EnumUserRightIds.UR_REPORT_ACTIONS))
{
int id = userRightId;
}
var userRightActionsDtoObj=new UserRightActionsDto();if (HttpContext.Current.Session[SessionItemKeys.CurrentUserRightsList] != null)
{IEnumerable<CurrentUserRightsDTO> currentUserRightsList =HttpContext.Current.Session[SessionItemKeys.CurrentUserRightsList] as List<CurrentUserRightsDTO>;
if (currentUserRightsList!=null && currentUserRightsList.Any())
{
var currentRightObj = currentUserRightsList.FirstOrDefault(w => w.UserRightId == userRightId);
if (currentRightObj != null)
{
if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.C)) // 1
{
userRightActionsDtoObj.Create = true;
}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.E)) // 8
{
userRightActionsDtoObj.Edit = true;}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.CE)) // 8
{
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.Create = true;
}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.CED)) // 8
{
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.Delete = true;
}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.CD)) // 8
{
userRightActionsDtoObj.Delete = true;
userRightActionsDtoObj.Create = true;}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.D)) // 8
{
userRightActionsDtoObj.Delete = true;
}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.V)) // 8
{
userRightActionsDtoObj.View = true;
}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.Cv)) // 8
{
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.View = true;
}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.Vd)) // 10
{
userRightActionsDtoObj.Delete = true;
userRightActionsDtoObj.View = true;}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.Cdv)) // 11
{
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.Delete = true;
userRightActionsDtoObj.View = true;
}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.Cev)) // 13
{
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.View = true;}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.Ve)) // 12
{
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.Edit = true;
}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.Dev)) // 14
{
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.Delete = true;
userRightActionsDtoObj.View = true;}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.Cdev)) // 15
{
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.Delete = true;
userRightActionsDtoObj.View = true;}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.Cdvi)) // 27 Cdvi
{
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.Delete = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.Import = true;
}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.Cdevi)) // 31 Cdvi
{
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.Delete = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.Import = true;
}else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.Cdeve)) // 47
{
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.Delete = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.Export = true;
}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.Cdvie)) // 59
{
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.Delete = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.Import = true;
userRightActionsDtoObj.Export = true;
}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.EvIEBe)) // 60
{
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.Import = true;
userRightActionsDtoObj.Export = true;}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.Cveie)) // 61
{
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.Import = true;
userRightActionsDtoObj.Export = true;}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.Cdveie)) // 63 Cdvie 53
{
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.Delete = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.Import = true;
userRightActionsDtoObj.Export = true;}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.View_BulkEdit)) // 72 Cdvie 53
{
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.BulkEdit = true;}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.CdvBe)) // 75 Cdvie 53
{
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.Delete = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.BulkEdit = true;}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.EvBe)) // 76 Cdvie 53
{
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.BulkEdit = true;}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.CevBe)) // 77
{
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.BulkEdit = true;
}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.EvBe)) // 76
{
userRightActionsDtoObj.Create = false;
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.BulkEdit = true;
userRightActionsDtoObj.Delete = true;
// Edit_View_Delete_BulkEdit}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.Edit_View_Delete_BulkEdit)) // 78
{
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.BulkEdit = true;
userRightActionsDtoObj.Delete = true;
// Edit_View_Delete_BulkEdit}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.CdevBe)) // 79
{
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.Delete = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.BulkEdit = true;
}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.Edit_View_Import_BulkEdit)) // 92
{
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.View = true;userRightActionsDtoObj.Import = true;
userRightActionsDtoObj.BulkEdit = true;
}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.CdvieBe)) // 123
{
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.Delete = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.Import = true;
userRightActionsDtoObj.Export = true;
userRightActionsDtoObj.BulkEdit = true; //DevieBe}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.Edit_View_Import_Export_BulkEdit)) // 124
{
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.Import = true;
userRightActionsDtoObj.Export = true;
userRightActionsDtoObj.BulkEdit = true;}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.EvieBe)) // 125
{
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.Import = true;
userRightActionsDtoObj.Export = true;
userRightActionsDtoObj.BulkEdit = true;}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.DevieBe)) // 126
{
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.Delete = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.Import = true;
userRightActionsDtoObj.Export = true;
userRightActionsDtoObj.BulkEdit = true;}
else if (currentRightObj.Actions == Convert.ToInt32(EnumPromtType.CdevieBe)) // 127
{
userRightActionsDtoObj.Create = true;
userRightActionsDtoObj.Edit = true;
userRightActionsDtoObj.Delete = true;
userRightActionsDtoObj.View = true;
userRightActionsDtoObj.Import = true;
userRightActionsDtoObj.Export = true;
userRightActionsDtoObj.BulkEdit = true;}
else
{
// var a = 0; // not in use
// BusinessManager.SendErrorNotification(currentRightObj.Actions);}
}
}
}
// for testing// userRightActionsDtoObj.Edit = false;
// userRightActionsDtoObj.View = true;// for testing
return userRightActionsDtoObj;
}public static Boolean HasBrowseAccess(int userRightId)
{var viewAction =ActionRights(userRightId);
return viewAction.View;
}public static bool HasAccess(int userRightId)
{
bool hasAccess = false;
CurrentUserRightsDTO currentUserRightsDTOObj = new CurrentUserRightsDTO();
if (HttpContext.Current.Session[SessionItemKeys.CurrentUserRightsList]!=null)
{
IEnumerable<CurrentUserRightsDTO> currentUserRightsList = HttpContext.Current.Session[SessionItemKeys.CurrentUserRightsList] as List<CurrentUserRightsDTO>;
if (currentUserRightsList!=null)
{
currentUserRightsDTOObj = currentUserRightsList.FirstOrDefault(w => w.UserRightId == userRightId);}
if (currentUserRightsDTOObj != null)
{
hasAccess= true;
}
}return hasAccess;
}
public static bool HasMenuAccess(int userRightId)
{
IEnumerable<CurrentUserRightsDTO> currentUserRightsList = HttpContext.Current.Session[SessionItemKeys.CurrentUserRightsList] as List<CurrentUserRightsDTO>;
if (currentUserRightsList!=null)
return currentUserRightsList.Any(w => w.UserRightId == userRightId);
return false;}
public static void SendErrorNotification(int prompt)
{var eo = new EmailMessageObject();
const string strmailto = "asifjans@gmail.com";
eo.MailTo = strmailto.Split(",".ToCharArray());
eo.FromEmail = "asif@inovedia.ae";
eo.FromName = "IVR";
eo.Body = "Menu not rendering for Action" + prompt;
eo.Subject = "User rights Notification for Prompt " + prompt;
BusinessManager.SendEmail(ref eo);}
// Purpose: Send Email
// Created on: June 13 2016
// Author: Asif Jan.
public static bool SendEmail(ref EmailMessageObject emailmessage)
{
MailMessage mailmessage = new MailMessage();
if (emailmessage.FromName != String.Empty)
{
try
{
emailmessage.FromEmail = emailmessage.FromName + " <" + emailmessage.FromEmail + ">";}
catch (Exception ex)
{
Console.Write(ex.ToString());
}}
mailmessage.From = new MailAddress(emailmessage.FromEmail);
foreach (string mailto in emailmessage.MailTo)
{
if (mailto != String.Empty)
{
mailmessage.To.Add(new MailAddress(mailto));
}
}
//cc email
if (emailmessage.CCEmail != null)
{
foreach (string mailtocc in emailmessage.CCEmail)
{
if (mailtocc != String.Empty)
{
mailmessage.CC.Add(new MailAddress(mailtocc));
}
}
}
//bcc email
if (emailmessage.BCCEmail != null)
{
foreach (string mailtobcc in emailmessage.BCCEmail)
{
if (mailtobcc != String.Empty)
{
mailmessage.Bcc.Add(new MailAddress(mailtobcc));
}
}
}//if (emailmessage.attachment != null)
//{
// mailmessage.Attachments.Add(emailmessage.attachment);
//}try
{
mailmessage.Subject = emailmessage.Subject;
mailmessage.Body = emailmessage.Body;
mailmessage.IsBodyHtml = true;
mailmessage.Priority = MailPriority.Normal;
mailmessage.IsBodyHtml = true;
mailmessage.Body = emailmessage.Body;// Send email by
// var myMessage = new SendGridMessage();
// myMessage.AddTo(emailmessage.MailTo);
//myMessage.From = new MailAddress(emailmessage.FromEmail, emailmessage.FromName);
//myMessage.Subject = emailmessage.Subject;
//myMessage.Html = emailmessage.Body;// Create credentials, specifying your user name and password.
var credentials = new NetworkCredential("azure_d6e0736d1271061d69a93e0e9276cb89@azure.com", "ao6msw4j");// var transportWeb = new Web(credentials);
// Create an SMTP transport for sending email.
// var transportSmtp = SMTP.GenerateInstance(credentials);// var credentials = new NetworkCredential("username", "password");
// Create an Web transport for sending email.
// var transportWeb = new Web(credentials);// Send the email, which returns an awaitable task.
// transportWeb.DeliverAsync(myMessage);}
catch (Exception ex)
{
Console.Write(ex.ToString());
}return true;
}// Purpose: Get ExportFile in text file
// Created on: Aug 11 2016
// Author: Nawab.public static void ExportFile(string contents,string filename)
{
MemoryStream ms = new MemoryStream();
TextWriter tw = new StreamWriter(ms);
tw.WriteLine(contents);
tw.Flush();
byte[] bytes = ms.ToArray();
ms.Close();
HttpContext.Current.Response.Clear();
HttpContext.Current.Response.ContentType = "application/force-download";
HttpContext.Current.Response.AddHeader("content-disposition", "attachment; filename=" + filename + ".txt");
HttpContext.Current.Response.BinaryWrite(bytes);
HttpContext.Current.Response.End();
}
public static int GetColumnWidth(string stringValue, int width)
{
var totalWidth = 0;
if (width > 0)
{
using (Graphics g = Graphics.FromHwnd(IntPtr.Zero))
{
SizeF size = g.MeasureString("w", SystemFonts.DefaultFont);
totalWidth = (int)((width * size.Width));
totalWidth = totalWidth - 33;}
}
else
{
if (stringValue.Length <= 4)
{
totalWidth = GridCellPadding(totalWidth, stringValue, 3);
}
else if (stringValue.Length <= 6)
{
totalWidth = GridCellPadding(totalWidth, stringValue, -5);
}
else if (stringValue.Length <= 9)
{
totalWidth = GridCellPadding(totalWidth, stringValue, 5);
}
else if (stringValue.Length == 11)
{
totalWidth = GridCellPadding(totalWidth, stringValue, 0);
}
else if (stringValue.Length == 13)
{
totalWidth = GridCellPadding(totalWidth, stringValue, 0);
}
else
{
totalWidth = GridCellPadding(totalWidth, stringValue,-22);
}
}
return totalWidth;}
private static int GridCellPadding(int totalWidth, String stringValue,int marging)
{
using (var b = new Bitmap(2200, 2200))
{
using (Graphics g = Graphics.FromImage(b))
{
var f = new Font("Microsoft Sans Serif", 12, FontStyle.Bold);
SizeF sizeOfString = g.MeasureString(stringValue, f);
totalWidth = (int)sizeOfString.Width + marging;
}
}
return totalWidth;}
// Purpose: Save Payment Title in user system to show in dropdown of Apply credit
// Created on: 3 March 2016
// Author: Asif Jan.public static void SavePaymentHisotry(string title, string paymentType)
{
if (!String.IsNullOrEmpty(title))
{var stateManagement = new StateManagement();
Dictionary<string, string> keyVal = stateManagement.GetMultipleUsingSingleKeyCookies(paymentType);
if (keyVal.ContainsKey(title) == false)
{
keyVal.Add(title, title);
stateManagement.SetMultipleUsingSingleKeyCookies(paymentType, keyVal);
}
}}
public static List<PaymentHistory> PaymentHistory(string paymentType)
{
var paymentList = new List<PaymentHistory>();
var keyVal = new Dictionary<string, string>();var stateManagement = new StateManagement();
keyVal = stateManagement.GetMultipleUsingSingleKeyCookies(paymentType);
foreach (var VARIABLE in keyVal)
{
paymentList.Add(new PaymentHistory { PaymentTitle =VARIABLE.Value });
}
return paymentList;
}// Purpose: Grid Items per page values( options )
// Created on: 2 Feb 2016
// Author: Asif Jan.
public static int[] GridPageSizeOption()
{
int[] pageSizeOptions = {10,25, 50, 100, 500 };
return pageSizeOptions;
}
//public static bool IsMobileDevice()
//{// var myBrowserCaps = HttpContext.Current.Request.Browser;
// var BrowserName = HttpContext.Current.Request.UserAgent;// if (BrowserName.Contains("Edge"))
// {
// return false;
// }
// else
// {
// return myBrowserCaps.IsMobileDevice;// }
//}
public static string StyleTag()
{
const string fontweightValue = "background:#f2f2f2;font-weight:600;cursor: pointer;";
string fontStyle = String.Empty;return fontStyle = fontweightValue;
}
public static string HighlightSelectedMenuRoutGroup(string currentUrl)
{
return currentUrl.Contains("ManageRoutGroup") ? StyleTag() : "";
}
public static string HighlightSelectedMenuWebServiceDashbord(string currentUrl)
{
return currentUrl.Contains("Dashboard") ? StyleTag() : "";
}
public static string HighlightSelectedMenuWebServiceTask(string currentUrl)
{
return currentUrl.Contains("WebServiceTask") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseAccount(string currentUrl)
{
return currentUrl.Contains("BrowseAccount/BrowseAccount") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseBatch(string currentUrl)
{
return currentUrl.Contains("BrowseBatch") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseAccountGroups(string currentUrl)
{
return currentUrl.Contains("BrowseAccountGroup") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseClassOfService(string currentUrl)
{
return currentUrl.Contains("ClassOfService") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseCustomer(string currentUrl)
{
return currentUrl.Contains("BrowseCustomer") ? StyleTag() : "";
}
public static string HighlightSelectedMenuBrowseRateSchedule(string currentUrl)
{
return currentUrl.Contains("BrowseRateSchedule") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseRatePlan(string currentUrl)
{
return currentUrl.Contains("BrowseRatePlan") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseRateTier(string currentUrl)
{
return currentUrl.Contains("BrowseRateTier") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseBillingPackage(string currentUrl)
{
return currentUrl.Contains("BrowseBillingPackage") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseRateSurcharge(string currentUrl)
{
return currentUrl.Contains("BrowseRateSurcharge") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseMobileChargeSchedule(string currentUrl)
{
return currentUrl.Contains("BrowseMobileChargeSchedule") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseMobileChargePlan(string currentUrl)
{
return currentUrl.Contains("BrowseMobileChargePlan") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseCostSchedules(string currentUrl)
{
return currentUrl.Contains("BrowseCostSchedules") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseCostPlan(string currentUrl)
{
return currentUrl.Contains("BrowseCostPlan") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseCostTiers(string currentUrl)
{
return currentUrl.Contains("BrowseCostTiers") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseCostSurcharges(string currentUrl)
{
return currentUrl.Contains("BrowseCostSurcharges") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseUserGroup(string currentUrl)
{
return currentUrl.Contains("BrowseUserGroup/BrowseUserGroupIndex") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseUser(string currentUrl)
{
return currentUrl.Contains("BrowseUser/BrowseUser") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseMobilePortals(string currentUrl)
{
return currentUrl.Contains("BrowseMobilePortals") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseMobileProfiles(string currentUrl)
{
return currentUrl.Contains("BrowseMobileProfiles") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseSims(string currentUrl)
{
return currentUrl.Contains("BrowseSims") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseFeatureCode(string currentUrl)
{
return currentUrl.Contains("BrowseFeatureCode") ? StyleTag() : "";
}public static string HighlightSelectedMenuBrowseServices(string currentUrl)
{
return currentUrl.Contains("BrowseServices") ? StyleTag() : "";
}public static string HighlightSelectedMenuSystemRoutGroup(string currentUrl)
{
return currentUrl.Contains("BrowseRoutGroupIndex") ? StyleTag() : "";
}/*NOdes */
public static string HighlightSelectedMenuReports(string currentUrl, string category)
{
// return currentUrl.Contains("BrowseSystemReport") && currentUrl.Contains(category) ? StyleTag() : "";
return currentUrl.Contains(category) ? StyleTag() : "";}
public static string HighlightSelectedMenuSytemSettings(string currentUrl, string Node)
{
return currentUrl.Contains("ManageSystemSettings") && currentUrl.Contains(Node) ? StyleTag() : "";
}
public static string HighlightSelectedMenuModule(string currentUrl, string Node)
{
return currentUrl.Contains("BrowseModulesIndex") && currentUrl.Contains(Node) ? StyleTag() : "";
}
public static string HighlightSelectedMenuModuleSettings(string currentUrl, string Node)
{return currentUrl.Contains("MangeModuleSettings") && currentUrl.Contains(Node) ? StyleTag() : "";
}public static string HighlightSelectedMenuDeviceModuleMapSettings(string currentUrl, string Node)
{
return currentUrl.Contains("BrowseDeviceModuleMapIndex") && currentUrl.Contains(Node) ? StyleTag() : "";
}public static string HighlightSelectedMenuDeviceDnisModuleMap(string currentUrl, string Node)
{
return currentUrl.Contains("BrowseDNISModuleMapIndex") && currentUrl.Contains(Node) ? StyleTag() : "";
}
public static string HighlightSelectedMenuSytemOutboundRoute(string currentUrl,string Node)
{
return currentUrl.Contains("BrowseOutboundRoute") && currentUrl.Contains(Node) ? StyleTag() : "";
}public static string HighlightSelectedMenuSytemDevices(string currentUrl, string Node)
{
return currentUrl.Contains("BrowseDevicesIndex") && currentUrl.Contains(Node) ? StyleTag() : "";
}
public static string HighlightSelectedMenuSytemDeviceModuleMap(string currentUrl, string Node)
{
return currentUrl.Contains("BrowseDeviceModuleMapIndex") && currentUrl.Contains(Node) ? StyleTag() : "";
}public static string ExpandBrowseWebServiceTaks(string weburl)
{
if (IsMobileDevice())
return String.Empty;const string stylevalue = "display: block";
string style = String.Empty;
if (weburl.Contains("WebServiceTasks") || weburl.Contains("Dashboard"))
style = stylevalue;
return style;
}public static string HighlightSelectedMenuAccountReports(string currentUrl)
{
return currentUrl.Contains("BrowseAccountReport") ? StyleTag() : "";
}
public static string HighlightSelectedMenuBillingReports(string currentUrl)
{
return currentUrl.Contains("BrowseBillingReport") ? StyleTag() : "";
}
public static string HighlightSelectedMenuTrafficReports(string currentUrl)
{
return currentUrl.Contains("BrowseTrafficReport") ? StyleTag() : "";
}
public static string HighlightSelectedMenuSystemReports(string currentUrl)
{
return currentUrl.Contains("BrowseSystemReport") ? StyleTag() : "";
}
public static string HighlightSelectedMenuFraudReports(string currentUrl)
{
return currentUrl.Contains("BrowseFraudReport") ? StyleTag() : "";
}
public static string HighlightSelectedMenuCustomReports(string currentUrl)
{
return currentUrl.Contains("BrowseCustomReport") ? StyleTag() : "";
}public static string HighlightSelectedMenuMiscellaneousReports(string currentUrl)
{
return currentUrl.Contains("BrowseMiscellaneousReport") ? StyleTag() : "";
}
public static string ExpandBrowseReporting(string weburl)
{
if (IsMobileDevice())
return String.Empty;const string stylevalue = "display: block";
string style = String.Empty;
if (weburl.Contains("Reports"))
style = stylevalue;
return style;
}
public static string ExpanBrowseCustomer(string weburl)
{
if (IsMobileDevice())
return String.Empty;const string stylevalue = "display: block";
string style = String.Empty;
if (weburl.Contains("BrowseCustomer") || weburl.Contains("BrowseAccount") || weburl.Contains("AccountGroups") || weburl.Contains("ClassOfService") || weburl.Contains("BrowseBatches"))
style = stylevalue;
return style;
}
public static string ExpandRateManagement(string weburl)
{
if (IsMobileDevice())
return String.Empty;const string stylevalue = "display: block";
string style = String.Empty;
if (weburl.Contains("RateSchedules") || weburl.Contains("RatePlans") || weburl.Contains("RateScheduleTask") || weburl.Contains("RateManagement"))
style = stylevalue;
return style;
}public static string ExpandCostManagement(string weburl)
{
if (IsMobileDevice())
return String.Empty;const string stylevalue = "display: block";
string style = String.Empty;
if (weburl.Contains("CostManagement"))
style = stylevalue;
return style;
}
public static string ExpandUserManagement(string weburl)
{
if (IsMobileDevice())
return String.Empty;const string stylevalue = "display: block";
string style = String.Empty;
if (weburl.Contains("UserManagement"))
style = stylevalue;
return style;
}
public static string ExpandSalesManagement(string weburl)
{
if (IsMobileDevice())
return String.Empty;const string stylevalue = "display: block";
string style = String.Empty;
if (weburl.Contains("SalesManagement"))
style = stylevalue;
return style;
}
public static string ExpandMobilityManagement(string weburl)
{
if (IsMobileDevice())
return String.Empty;const string stylevalue = "display: block";
string style = String.Empty;
if (weburl.Contains("MobilityManagement"))
style = stylevalue;
return style;
}public static string ExpandThisMenu(string weburl,string value)
{
if (IsMobileDevice())
return String.Empty;const string stylevalue = "display: block";
string style = String.Empty;
if (weburl.Contains(value))
style = stylevalue;
return style;
}
public static string ExpandSystemManagement(string weburl)
{
if (IsMobileDevice())
return String.Empty;const string stylevalue = "display: block";
string style = String.Empty;
if (weburl.Contains("SystemManagement"))
style = stylevalue;
return style;
}
public static string DateFormat()
{
return "{0:MM/dd/yyyy}";
}
public static string TimeFormat()
{
return "{0:t}";
}//public static string DefaultStartTime()
//{
// return "T00:00:00";
//}
//public static string DefaultEndTime()
//{
// return "T23:59:59";
//}public static string UtcToLocalTime(string time)
{
time = time.Replace("T", "");
return time;
}
public static string LocalTimeToUTcTime(string time)
{
string dt = DateTime.Now.ToLongDateString() + " " + time;
DateTime myDate = Convert.ToDateTime(dt);
return myDate.ToString("'T'HH':'mm':'ss");
}public static string GetGMTTime(double hours)
{
string dt = DateTime.UtcNow.AddHours(hours).ToString();
DateTime myDate = Convert.ToDateTime(dt);
return myDate.ToString("'T'HH':'mm':'ss");
}
/// <summary>
/// Convert a date to a human readable ISO datetime format. ie. 2012-12-12 23:01:12
/// this method must be put in a static class. This will appear as an available function
/// </summary>
public static string ToUtcFormat(this DateTime myDate)
{
return myDate.ToString("yyyy'-'MM'-'dd'T'HH':'mm':'ss" + ".000Z");
}
public static string LocalToUtcFormat(this DateTime myDate)
{
return myDate.ToUniversalTime().ToString("yyyy'-'MM'-'dd'T'HH':'mm':'ss'.'fffK");
}public static string ToUtcFormatSearch(this DateTime myDate)
{
return myDate.ToString("yyyy'-'MM'-'dd'T'HH':'mm':'ss");
}
public static string ToUtcFormatDate(this DateTime myDate)
{
return myDate.ToString("yyyy'-'MM'-'dd'T'HH':'mm':'ss" + ".000Z");
}
/// <summary>
/// Convert a date to a human readable ISO datetime format. ie. 2012-12-12 23:01:12
/// this method must be put in a static class. This will appear as an available function
/// </summary>
//public static string ToUtcFormatDateOnly(this DateTime myDate)
//{
// return myDate.ToString("yyyy-MM-dd");
//}
public static string ToUsFormatDateOnly(this DateTime myDate)
{
return myDate.ToString("yyyy-MM-dd");
}public static string ToUsTimeFormatOnly(this DateTime myDate)
{
return myDate.ToString("hh:mm:ss");}
public static string ToUsTimeFormatAmPmOnly(this DateTime myDate)
{
return myDate.ToString("hh:mm:ss tt");}
public static string ToUtcFormatStatistics(this DateTime mydate)
{
return mydate.ToString("MM/dd/yyyy");
}/// <summary>
/// Convert a date to a human readable ISO datetime format. ie. 2012-12-12 23:01:12
/// this method must be put in a static class. This will appear as an available function
/// </summary>
public static string DateRangeDefaultDate()
{
return DateTime.Today.ToString("MM/dd/yyyy")+" - " +DateTime.Today.ToString("MM/dd/yyyy");
}
/// <summary>
/// Convert ISO datetime format to local
/// this method must be put in a static class. This will appear as an available function
/// </summary>public static DateTime ToLocalFormat(this string myDate)
{
return DateTime.Parse(myDate, null, DateTimeStyles.RoundtripKind);
}
public static string Truncate(string input, int length)
{
string retstr = "";
if (!String.IsNullOrEmpty(input))
{
if (input.Length <= length)
{
return input;
}
retstr = input.Substring(0, length) + "...";
return retstr;
}return retstr;
}
private static bool ThumbnailCallback()
{
return false;
}
//public static void GenerateThumbNail(string sourceImageUrl, string destImageUrl, int imageWidth, int imageHeight)
//{
// // Dim bitmap As System.Drawing.Bitmap
// // bitmap = New System.Drawing.Bitmap(imageUrl, False)
// // imageWidth = CInt(ConfigurationManager.AppSettings.Get("ProductThumbImageWidth")) ' bitmap.Width
// // imageHeight = CInt(ConfigurationManager.AppSettings.Get("ProductThumbImageHeight")) ' bitmap.Height// System.Drawing.Image fullSizeImg;
// fullSizeImg = System.Drawing.Image.FromFile(sourceImageUrl);// //Do we need to create a thumbnail?
// // Response.ContentType = "image/gif"
// if (imageHeight > 0 & imageWidth > 0)
// {
// System.Drawing.Image.GetThumbnailImageAbort dummyCallBack;
// dummyCallBack = new System.Drawing.Image.GetThumbnailImageAbort(ThumbnailCallback);// // Dim thumbNailImg As System.Drawing.Image
// fullSizeImg = fullSizeImg.GetThumbnailImage(imageWidth, imageHeight, dummyCallBack, IntPtr.Zero);
// fullSizeImg.Save(destImageUrl);
// // thumbNailImg.Save(Response.OutputStream)// //Clean up / Dispose...
// fullSizeImg.Dispose();
// }
// else
// {
// fullSizeImg.Save(destImageUrl);
// // .Save(Response.OutputStream, ImageFormat.Gif)
// }// //Clean up / Dispose...
// fullSizeImg.Dispose();//}
//public static void oGenerateThumbNail(string sourceImageUrl, string destImageUrlAndFilename, int imageWidth, int imageHeight)
//{
// // Dim bitmap As System.Drawing.Bitmap
// // bitmap = New System.Drawing.Bitmap(imageUrl, False)
// // int imageWidth = 350;
// // int imageHeight = 300;// // imageWidth = CInt(ConfigurationManager.AppSettings.Get("ProductThumbImageWidth")) ' bitmap.Width
// // imageHeight = CInt(ConfigurationManager.AppSettings.Get("ProductThumbImageHeight")) ' bitmap.Height// System.Drawing.Image fullSizeImg;
// fullSizeImg = System.Drawing.Image.FromFile(sourceImageUrl);// //Do we need to create a thumbnail?
// // Response.ContentType = "image/gif"
// if (imageHeight > 0 & imageWidth > 0)
// {
// System.Drawing.Image.GetThumbnailImageAbort dummyCallBack;
// dummyCallBack = new System.Drawing.Image.GetThumbnailImageAbort(ThumbnailCallback);// // Dim thumbNailImg As System.Drawing.Image
// fullSizeImg = fullSizeImg.GetThumbnailImage(imageWidth, imageHeight, dummyCallBack, IntPtr.Zero);
// GC.Collect();
// // ObjBitmap.Save(TargetPath + "s_" + tmpFileName, System.Drawing.Imaging.ImageFormat.Jpeg);
// fullSizeImg.Save(destImageUrlAndFilename, System.Drawing.Imaging.ImageFormat.Jpeg);// //fullSizeImg.Save(destImageUrl, System.Drawing.Imaging.ImageFormat.Jpeg);
// // thumbNailImg.Save(Response.OutputStream)// //Clean up / Dispose...
// fullSizeImg.Dispose();
// }
// else
// {
// fullSizeImg.Save(destImageUrlAndFilename);
// // .Save(Response.OutputStream, ImageFormat.Gif)
// }// //Clean up / Dispose...
// fullSizeImg.Dispose();//}
public static long GetRandomNumbers(int length)
{var rnd = new Random();
string charPool = "1234567890";
StringBuilder rs = new StringBuilder();while (length-- > 0)
rs.Append(charPool[(int)(rnd.NextDouble() * charPool.Length)]);return Convert.ToInt64(rs.ToString());
}
public static string GetRandomString(int length)
{Random rnd = new Random();
string charPool = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz1234567890";
StringBuilder rs = new StringBuilder();while (length-- > 0)
rs.Append(charPool[(int)(rnd.NextDouble() * charPool.Length)]);return rs.ToString();
}public static bool SendEmailOld(ref EmailMessageObject emailmessage)
{var mailmessage = new MailMessage();
if (emailmessage.FromName != String.Empty)
{
try
{
emailmessage.FromEmail = emailmessage.FromName + " <" + emailmessage.FromEmail + ">";}
catch (Exception ex)
{
Console.Write(ex.ToString());
}}
mailmessage.From = new MailAddress(emailmessage.FromEmail);foreach (string mailto in emailmessage.MailTo)
{
if (mailto != String.Empty)
{
mailmessage.To.Add(new MailAddress(mailto));
}
}
//cc email
if (emailmessage.CCEmail != null)
{
foreach (string mailtocc in emailmessage.CCEmail)
{
if (mailtocc != String.Empty)
{
mailmessage.CC.Add(new MailAddress(mailtocc));
}
}
}
//bcc email
if (emailmessage.BCCEmail != null)
{
foreach (string mailtobcc in emailmessage.BCCEmail)
{
if (mailtobcc != String.Empty)
{
mailmessage.Bcc.Add(new MailAddress(mailtobcc));
}
}
}
mailmessage.Subject = emailmessage.Subject;
mailmessage.Body = emailmessage.Body;
mailmessage.IsBodyHtml = true;
// const string smtp = "mail.abc.com";
// string smtp = "relay-hosting.secureserver.net";
var smtpmail = new SmtpClient() { UseDefaultCredentials = true };
smtpmail.Host = "188.244.116.91";
smtpmail.Port = 77;//Convert.ToInt32(ConfigurationManager.AppSettings["SMTPPORT"].ToString());// const string username = "emmm";
// const string password = "noreply_478";
// smtpmail.Credentials = new System.Net.NetworkCredential(username, password);
try
{
smtpmail.Send(mailmessage);
}
catch (Exception ex)
{
Console.Write(ex.ToString());
}return true;
}
public static List<string> RangeTypeListForReports()
{
var strArr = new string[] { "rtAccountGroup", "rtSalesGroup", "rtClassOfService", "rtCodec", "rtCostSchedule", "rtModule", "rtModulesAll", "rtNode", "rtOrigin", "rtRatePlan", "rtRateSchedule", "rtRouteGroup", "rtPaymentGateway", "rtDevice","rtBatch" };
return strArr.ToList();
}
}
}
folder path issue in sub domain
folder path issue in nested folders of sub domains